How to Sleep for X Seconds in Ruby
Stewart Nguyen
Feb 02, 2024
Ruby
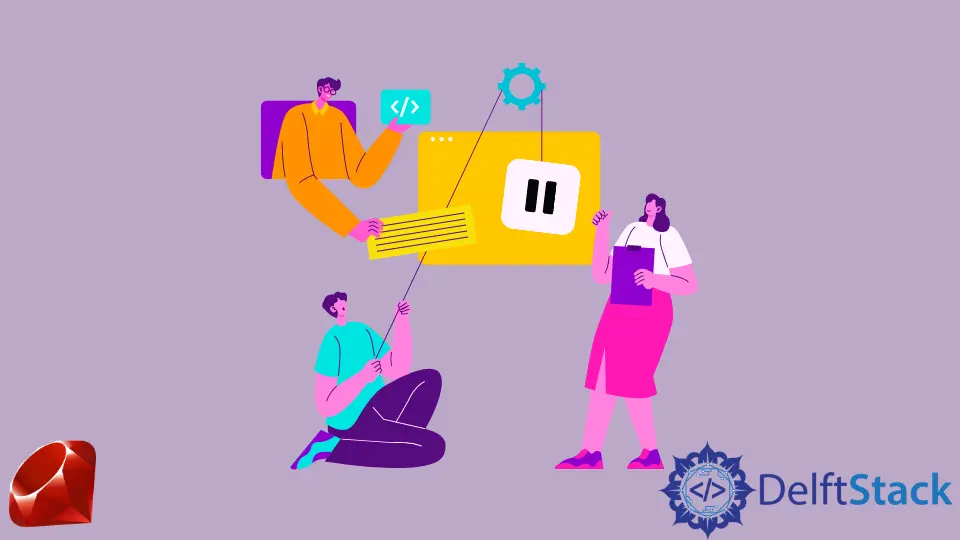
In some cases, we may want to pause the execution for some time; this is useful when we need to reduce the throughput of incoming background jobs or when we need to overcome the external API rate limit, etc.
We can use ruby’s sleep
method to suspend the execution in such cases.
The method sleep
takes several seconds as an argument and suspends the program for that number of seconds.
It will never stop if you call sleep
without any arguments.
start = Time.new
sleep 2
puts (Time.new - start).round
Output:
2
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe