Array.shift() in Ruby
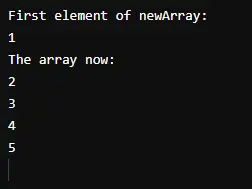
This article will discuss what Array.shift()
is and for what purposes we can use it in Ruby.
Array.shift()
in Ruby
Arrays are the most important part of any programming language. Arrays are used to store multiple data in a single variable based on keys or based on simple indexes.
Arrays store data based on the data types. We can store numbers, alphabets, strings, images, and objects in them.
There are many methods in Ruby that can be used with arrays. Array.shift()
is one of those methods.
It’s used to get the first value of the array and remove it from the array simultaneously. Let’s go through a basic example in which we will create a new array of numbers and use the .shift()
method to pop out the first element of that array.
The example is shown below.
newArray = [1, 2, 3, 4, 5]
puts 'First element of newArray:'
puts newArray.shift
puts 'The array now:'
puts newArray
The output of the above example is shown below.
As seen from the above example, by using the .shift()
method, we have popped the array’s first element, and the rest of the array is also displayed.
Let’s imagine if now we want to pop out many elements from the start of the array. We can do it easily by passing the number of elements we want to pop out from the array.
Let’s go through another example where we will pop several elements from different locations.
We will also include some nil
values inside our arrays to check the response of Ruby’s array.shift()
method. The example is shown below.
firstArray = [1, 2, 3, 4, 5]
secondArray = ['a', 'b', 'c', nil]
thirdArray = %w[abc def ghi]
puts 'First element of firstArray:'
puts firstArray.shift
puts 'Last element of secondArray:'
puts secondArray.shift(4)
puts 'Second element of thirdArray:'
puts thirdArray.shift(2)
The output of the above example is shown below.
As seen from the above example, by passing the number of items we want to pop out from the array, we can pop out the same amount of elements from the arrays.