The send Method in Ruby
-
Call a Method Without the
send
Method in Ruby -
Use the
send
Method to Invoke Private Method in Ruby -
Use the
send
Method to Invoke Method at Runtime in Ruby
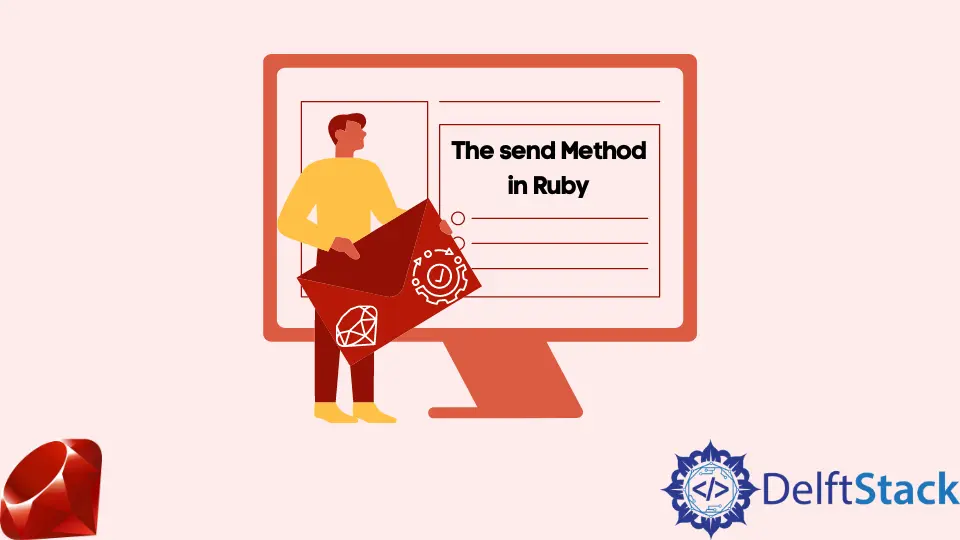
In Ruby, there are several ways to call a method. This article will discuss the use of the send
method and its benefits.
Call a Method Without the send
Method in Ruby
Usually, we would use .
followed by the method name to call a method on an object.
Consider the following example:
[1, 2, 3].join
=> "123"
The method join
is called on the array [1, 2, 3]
in the code snippet above. Using .
only works on the object’s public methods; calling a private method is impossible.
Use the send
Method to Invoke Private Method in Ruby
To call a private method, use the send
method. Consider the following code snippet.
class Foo
private
def secret
'secret that should not be told'
end
def my_asset(amount, location)
"I have #{amount}$ in #{location}"
end
end
Foo.new.secret
Foo.new.my_asset(100, 'the basement')
Output:
NoMethodError: private method 'secret' called for #<Foo>
NoMethodError: private method 'my_asset' called for #<Foo>
We must use the send
method to call the methods defined under the private
keyword.
Foo.new.send(:secret)
Foo.new.send(:my_asset, 100, 'the basement')
Output:
=> "secret that should not be told"
=> "I have 100$ in bank"
The send
method’s first argument is the method name, which can be a string or a symbol. The origin method’s arguments are also accepted via the second and third arguments, and so on.
Use the send
Method to Invoke Method at Runtime in Ruby
When there is a variable that stores the method name, send
comes in handy.
Example:
class Report
def format_xml
'XML'
end
def format_plain_text
'PLAIN TEXT'
end
end
def get_report_format(extension)
if extension == 'xml'
'format_xml'
else
'format_plain_text'
end
end
report_format = get_report_format('xml')
report = Report.new
formated_report = report.send(report_format)
Output:
"XML"
The class Report
is responsible for generating the format in the example above. It supports format_xml
and format_plain_text
.
We also have a method called get report_format
, which returns the format that Report
supports.
We can’t use the .
notation to call format_xml
directly. Instead, we must use the send
function: report.send(report format)
.