require vs include in Ruby
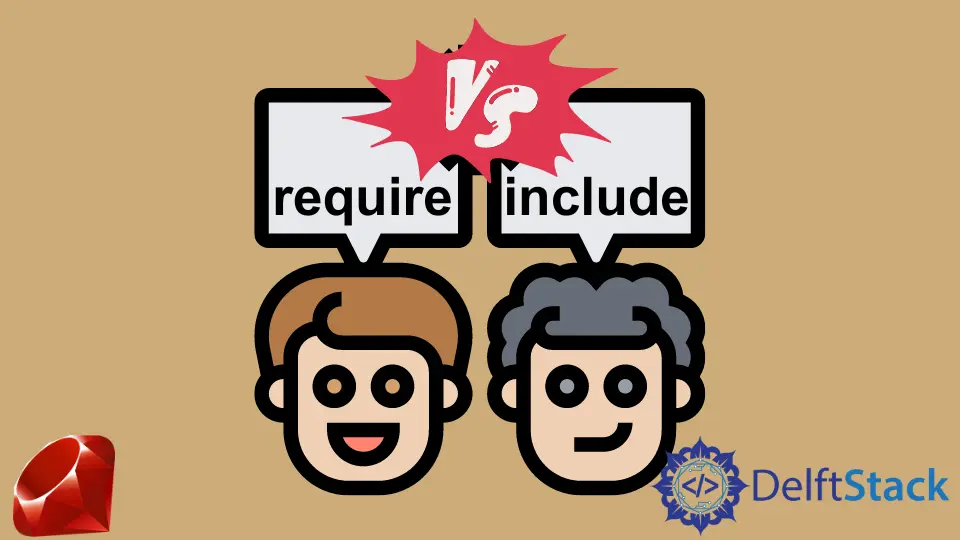
This article will clarify the differences between require and include in Ruby.
To achieve reusability and make maintenance more accessible, we must divide our features into files and modules.
Programmers can define as many modules as they want within each file.
Use the require
Method in Ruby
The file name is passed as a string to the require
method. It can be a path to a file, such as ./my dir/file a.rb
or a simple filename without an extension, such as file a.rb.
When you use require,
the code in a file is evaluated and executed.
The example shows how to use require
. The message in file a.rb
will be printed by the file code in file a.rb
.
We’ll load file_a
by calling require 'file_a'
inside file file b.rb
. Result in a string in file_a.rb
will be printed.
rubyCopy# file_a.rb
puts 'in file_a.rb'
# file_b.rb
require 'file_a'
Output:
textCopyin file_a.rb
Use the include
Method in Ruby
Unlike require
, which loads an entire file’s code, include
takes a module name and makes all its methods available to other classes or modules.
Below is the syntax for the include statement in Ruby. When we call the instance method greet
from a class called HelloWorld
, we get a missing error.
rubyCopyclass HelloWorld; end
HelloWorld.new.greet
Output:
textCopyNoMethodError: undefined method `greet' for #<HelloWorld:0x007faa8405d5a8>
Then we make a Greeting
module and include it in the class we created earlier. Calling greet
after that will print a message.
rubyCopymodule Greeting
def greet
puts 'How are you doing?'
end
end
HelloWorld.include(Greeting)
HelloWorld.new.greet
Output:
textCopyHow are you doing?