How to Read Lines of a Files in Ruby
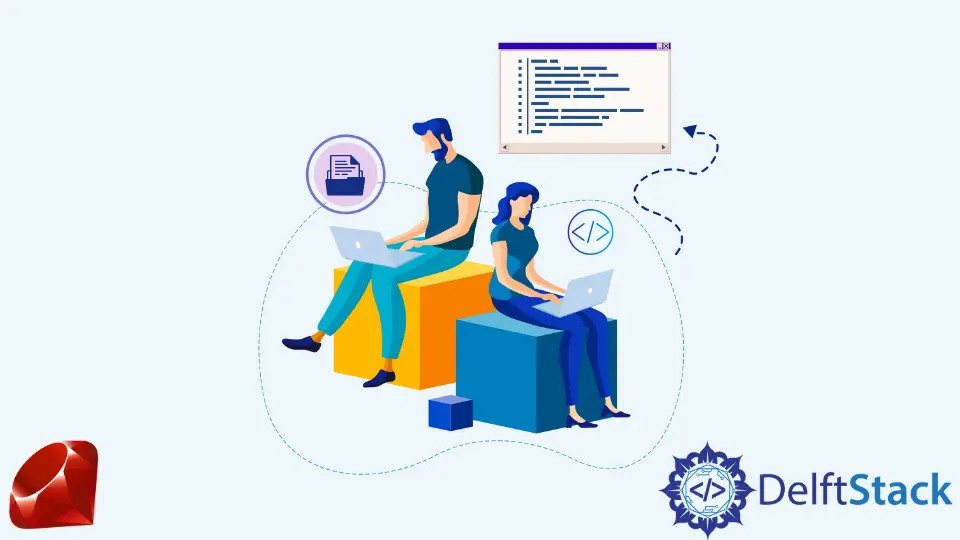
In Ruby, a File
object is an abstraction for any file that the current program can access.
We need to work with files for various reasons, including exporting monthly reports in CSV format, reading data files, and importing them into our database.
This post will look at two different ways to read a file in Ruby.
Use File#readlines
to Read Lines of a File in Ruby
File#readlines
takes a filename to read and returns an array of lines. Newline character \n
may be included in each line.
lines = File.readlines('foo')
Output:
["line 1\n", "line 2"]
We must be cautious when working with a large file, File#readlines
will read all lines at once and load them into memory.
Use File#foreach
to Read Lines of a File in Ruby
File#foreach
takes a filename and a block that specifies how it should process the current line. foreach
is superior to readlines
because it reads line by line; hence it uses less memory.
File.foreach('foo') do |line|
puts line
end
Output:
line 1
line 2