How to Read Files in Ruby
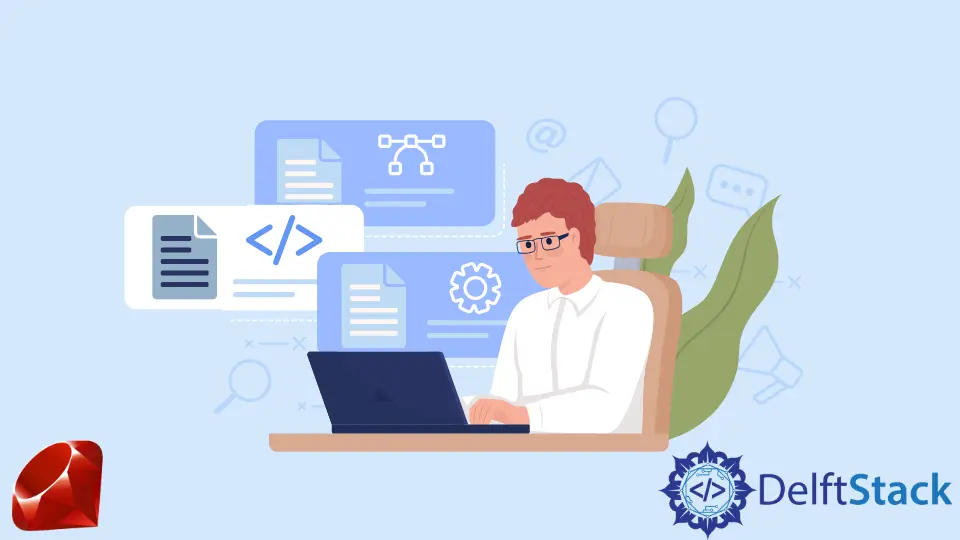
This article will teach us how to read Ruby files using different methods.
Read Files in Ruby
When we talk about high-level programming languages, Ruby is one of them, and it is also an interpreter. By using this language, we can use various programming paradigms.
Ruby programming language involves data types, and everything is an object. Static websites, data processing languages to automate tools, and desktop applications are also built using the Ruby programming language.
While working on applications, there are some situations in which we may need to open and read files in our applications. A few methods can be used to read files in Ruby.
The simple process of reading a file is to open a file using the File
method, and then by using the various read
methods, we can read the files. There are two methods in Ruby.
If we want to read files line by line, we can use the readlines
method. It is a very simple and easy-to-use method.
Let’s go through an example and try to open and read the file, as shown below.
# Ruby
openFile = File.open('data.txt')
puts openFile.readlines
Output:
Moreover, we can use the read
method to read full files. It is a very simple and easy-to-use method.
Let’s go through an example and try to open and read the file, as shown below.
# Ruby
openFile = File.open('data.txt')
puts openFile.read
Output: