How to Parse XML With Ruby
- What is Nokogiri?
- Parsing XML Files with Nokogiri
- Extracting Attributes from XML
- Modifying XML Files
- Conclusion
- FAQ
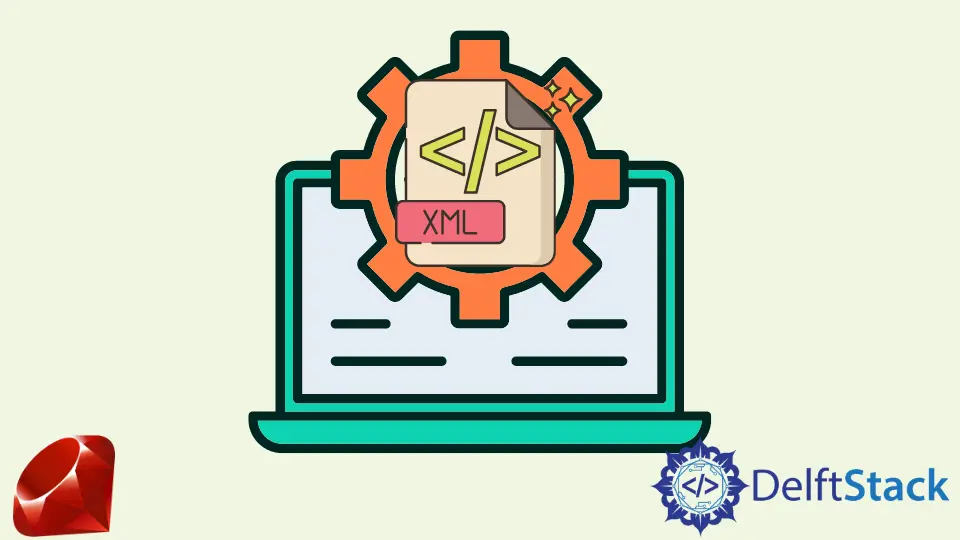
Parsing XML files can be an essential task for developers, especially when dealing with data interchange between systems. If you’re a Ruby enthusiast, you’re in luck! This article will guide you through the process of parsing XML using the powerful Nokogiri gem. Nokogiri is known for its speed and ease of use, making it a favorite among Ruby developers. Whether you’re extracting data from an XML file or transforming it, you’ll find that Nokogiri simplifies the task significantly. So, let’s dive into the world of XML parsing with Ruby and discover how to make the most of this robust library.
What is Nokogiri?
Nokogiri is a Ruby gem that provides an easy way to parse XML and HTML documents. It is built on top of the libxml2 library, which means it’s incredibly fast and efficient. With Nokogiri, you can search documents using XPath or CSS selectors, manipulate XML structures, and even create new XML documents from scratch. Installing Nokogiri is straightforward, and once you have it set up, you’ll be ready to parse XML files in no time.
To install Nokogiri, you can use the following command in your terminal:
gem install nokogiri
Now that you have Nokogiri installed, let’s explore how to parse XML files effectively.
Parsing XML Files with Nokogiri
Parsing an XML file using Nokogiri is quite simple. The first step is to load the XML file into a Nokogiri document. Once loaded, you can navigate through the XML structure and extract the data you need.
Here’s a basic example of how to parse an XML file:
require 'nokogiri'
require 'open-uri'
xml_file = 'path/to/your/file.xml'
doc = Nokogiri::XML(File.open(xml_file))
doc.xpath('//element_name').each do |element|
puts element.text
end
In this code, we first require the necessary libraries. We then specify the path to the XML file we want to parse. The Nokogiri::XML
method loads the XML file into a document object. The xpath
method allows us to search for specific elements within the XML. In this case, we are looking for elements named element_name
. For each found element, we print its text content.
Output:
Example output of the parsed element text
This example demonstrates the basic structure for parsing XML with Nokogiri. You can easily modify the XPath expression to target different elements or attributes within your XML file.
Extracting Attributes from XML
In addition to retrieving text content, you might want to extract attributes from XML elements. Nokogiri makes this straightforward as well. Let’s look at an example where we extract attributes from XML elements.
require 'nokogiri'
require 'open-uri'
xml_file = 'path/to/your/file.xml'
doc = Nokogiri::XML(File.open(xml_file))
doc.xpath('//element_name').each do |element|
puts element['attribute_name']
end
In this snippet, we are still using the same XML file. However, instead of printing the text content of the element_name
, we are accessing an attribute called attribute_name
using square brackets. This allows us to retrieve specific attributes associated with each element.
Output:
Example output of the extracted attribute value
This method is particularly useful when you need to gather metadata or additional information stored as attributes in your XML structure.
Modifying XML Files
Nokogiri also enables you to modify XML files easily. You can add, update, or remove elements and attributes as needed. Here’s how you can modify an existing XML document.
require 'nokogiri'
require 'open-uri'
xml_file = 'path/to/your/file.xml'
doc = Nokogiri::XML(File.open(xml_file))
new_element = Nokogiri::XML::Node.new('new_element', doc)
new_element.content = 'This is a new element'
doc.root.add_child(new_element)
File.write(xml_file, doc.to_xml)
In this example, we load the XML file just like before. We create a new XML node named new_element
and set its content. Then, we add this new element as a child to the root of the document. Finally, we write the modified document back to the file.
Output:
The XML file has been updated with the new element.
This capability allows you to dynamically change your XML documents based on your application’s requirements, making Nokogiri a powerful tool for XML manipulation.
Conclusion
Parsing XML with Ruby using the Nokogiri gem is an efficient and straightforward process. Whether you’re extracting data, modifying documents, or working with attributes, Nokogiri provides a robust set of features that simplify XML handling. With just a few lines of code, you can navigate complex XML structures and perform various operations. As you continue to explore the capabilities of Nokogiri, you’ll find that it’s an invaluable asset in your Ruby toolkit.
FAQ
-
What is Nokogiri?
Nokogiri is a Ruby gem that helps in parsing XML and HTML documents efficiently. -
How do I install Nokogiri?
You can install Nokogiri by running the commandgem install nokogiri
in your terminal. -
Can I modify XML files using Nokogiri?
Yes, Nokogiri allows you to add, update, or remove elements and attributes in XML files. -
What is the difference between XPath and CSS selectors in Nokogiri?
XPath is a language for navigating XML documents, while CSS selectors are used to select elements based on their attributes and hierarchy. Both can be used in Nokogiri to query XML. -
Is Nokogiri fast?
Yes, Nokogiri is built on the libxml2 library, making it one of the fastest options available for parsing XML in Ruby.