The not Keyword in Ruby
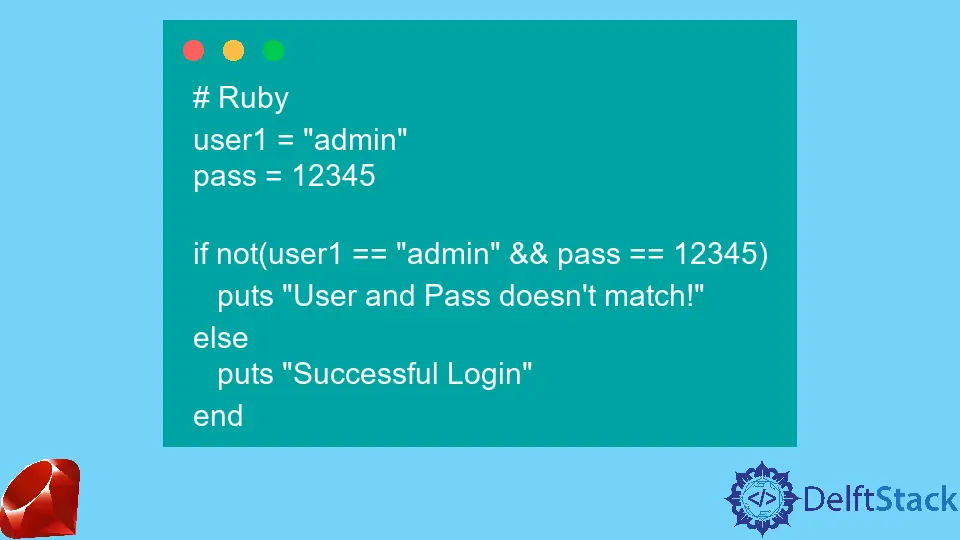
We will introduce the not
keyword in Ruby with examples.
the not
Keyword in Ruby
If we want to get an expression and then switch its boolean value, we use the not
keyword. If we want to get the expression that is normally returning a true as a result and we want it to return a false, we can use the not
keyword before the expression.
The syntax of the not
keyword is shown below.
# ruby
a = 5;
if not a < 7
puts "False"
end
The not
keyword works like Ruby’s !
operator. The only difference between them is that the !
operator has the most elevated importance of all operators, and the not
operator has the most down.
Now, let’s take different examples and see how to use the not
keyword in Ruby. Here is an example.
# Ruby
username = 'admin'
if username != 'Admin'
puts 'Incorrect username!'
else
puts 'Welcome, Admin!'
end
Output:
As we can see from the above example, the above code has returned the incorrect password because it is case-sensitive. Now, let’s take a little more complicated code of the not
operator in Ruby.
Consider the code as shown below.
# Ruby
user1 = 'admin'
pass = 12_345
if not ((user1 == 'admin' && pass == 12_345))
puts "User and Pass doesn't match!"
else
puts 'Successful Login'
end
On execution, it will produce the following output:
As we can see from the above example, the statement returned true, and then the not
keyword flipped the result. The output was printed from the else
statement.
In this way, we can use the not
keyword in our code in Ruby for multiple tasks where we want to flip the returned value from true to false or false to true.