nil, empty and blank in Ruby
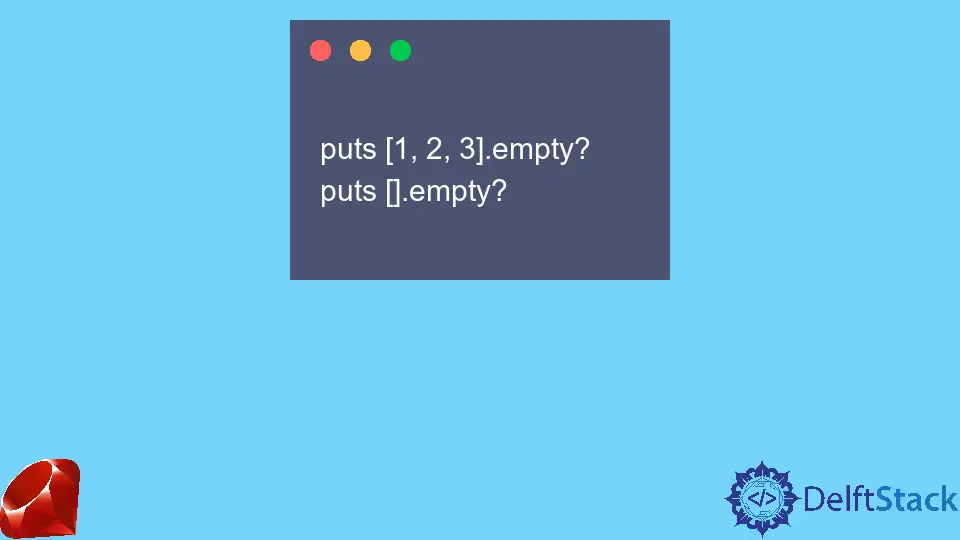
Sometimes we need to confirm whether the array is empty or filled with data. Or we may need to know the properties of the array.
In Ruby, there are some keywords available for this purpose.
In this article, we will see the use of nil
, empty
, and blank
in Ruby and some examples to make the topic easier.
The keyword nil
, empty
, and blank
are the built-in keywords that can be used for this purpose. Let’s discuss them in detail.
Use the nil
Keyword in Ruby
nil
is similar to null
in other programming languages. The keyword nil
means nothing or there is some absence.
By using the keyword nil
, you can check whether a value is nil
.
In our example below, we will see how we can use the nil
keyword in Ruby. Let’s take a look at the below example code.
array = [1, 2, 3, 4, 5]
puts array[5].nil?
Now the program above will show you the below output.
true
Use the empty
Keyword in Ruby
empty
is a built-in keyword in Ruby that allows you to check an array if it’s empty.
In our example below, we will see the use of empty
. Look at the below example code.
puts [1, 2, 3].empty?
puts [].empty?
Now the program above will show you the below output.
false
true
Use the blank
Keyword in Ruby
blank
is a built-in keyword in Ruby that allows you to check an array if it’s blank. It is mostly similar to empty
.
In our example below, we will see the use of blank
. Look at the below example code.
puts [].blank?
Now the program above will show you the below output.
true
The keyword nil
is used in objects, and it will return true
if the object is nil
, whereas empty
is mainly used on strings, hashes, and arrays, and it will return true
if the length of the string or array or hash is 0
. And the blank
is similar to empty
; the only difference is that it is implemented by Ruby Rails.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn