How to Create Multi-Line Comment in Ruby
Stewart Nguyen
Feb 02, 2024
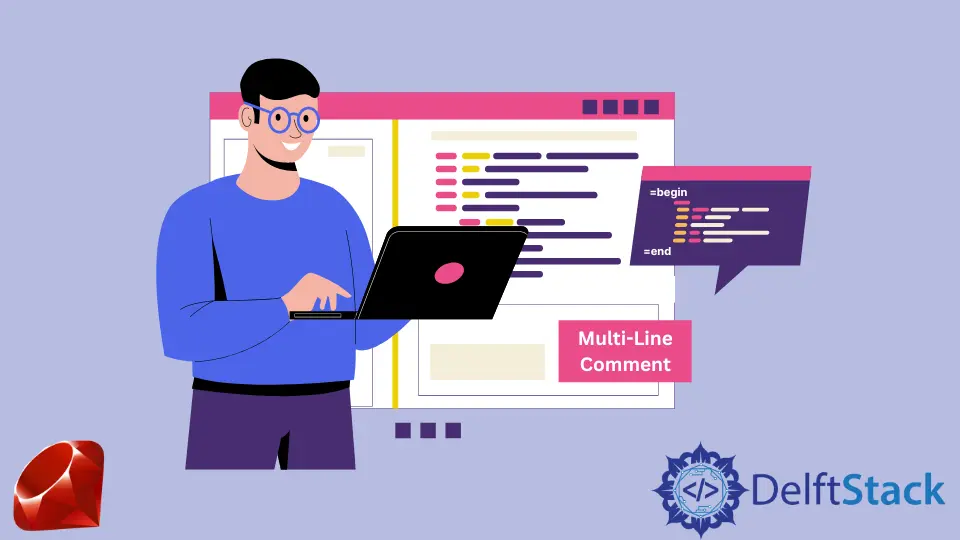
Like all other programming languages, Ruby also supports comment syntax out of the box.
We may use the number sign character #
to make a single-line comment in Ruby code.
Multi-Line Comment in Ruby
In Ruby, multi-lines comment syntax starts with the =begin
token and ends with the =end
token. It’s known as a block comment.
There should be no leading whitespace in these tokens.
# Quisque velit nisi, pretium ut lacinia in, elementum id enim.
# Praesent sapien massa, convallis a pellentesque nec, egestas non nisi.
# Donec rutrum congue leo eget malesuada.
# Curabitur arcu erat, accumsan id imperdiet et, porttitor at sem.
Although Ruby has the =begin
/=end
syntax for making multi-line comments, most Ruby developers favor single-line syntax, which can span multiple lines and is recommended.
# This is also
# multi-line comments