How to Generate Random Number in Ruby
-
Use the
rand
Method to Generate Random Numbers in Ruby -
Use the
Array.sample
Method to Generate Random Numbers in Ruby
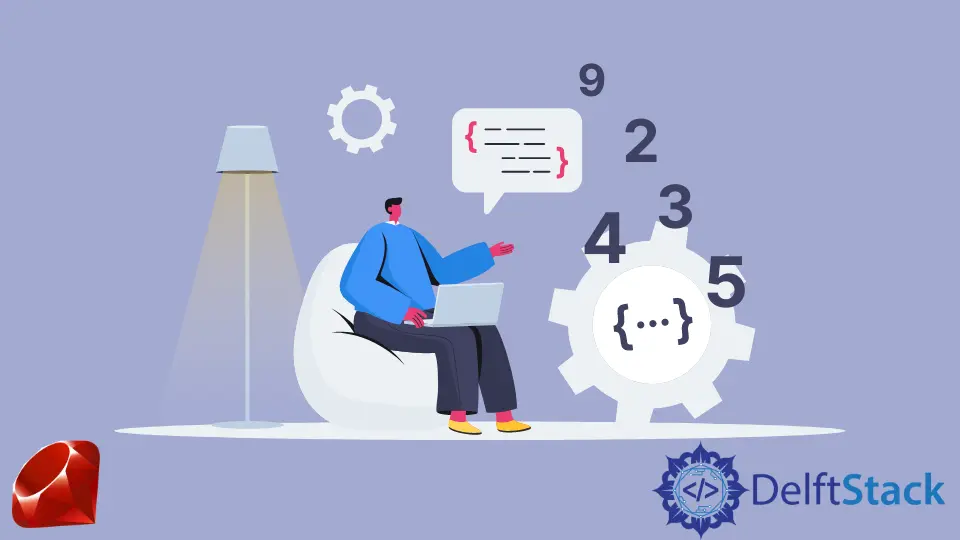
We’ll go over some methods for generating random numbers in Ruby in this article.
Use the rand
Method to Generate Random Numbers in Ruby
The rand
method generates a random number in Ruby. If there is no argument, the range will be 0
to 1
.
The method rand
takes an integer or a range as an argument. Take note that the outputs of the following examples are different in every run.
Example:
rand
Output:
0.6904532039551833
A value that is greater than 0
and less than 1
will be returned if rand
is called without an argument. We can pass the argument to the function call to change the range.
Example:
rand(10)
Output:
7
In this example, rand(10)
returns a random number between 0 and 9 (inclusive).
Example:
rand(10..20)
Output:
11
If you pass a range object to rand()
, it will return a number between 10
and 20
(inclusive). Use three(3) dot range 10...20
to exclude the maximum value.
Use the Array.sample
Method to Generate Random Numbers in Ruby
Array#sample
is another way to generate random numbers. To return a random number between 0
and 9
.\
Example:
(0..9).to_a.sample
Output:
5
We can specify the maximum digits by providing an integer to the sample
method, followed by Array.join
. The result is a string.
Example:
(0..9).to_a.sample(4).join
Output:
"6349"
The following is what the code example above will do.
- Generate a range object from 0 to 9.
- Convert that range object to an array using
Range#to_a
. - Return 4 random elements.
- Concatenate the elements in that array together using
Array#join
.