How to Find Array Index in Ruby
- Using the Index Method
- Using the Each_with_index Method
- Using the Find_index Method
- Using the Rindex Method
- Conclusion
- FAQ
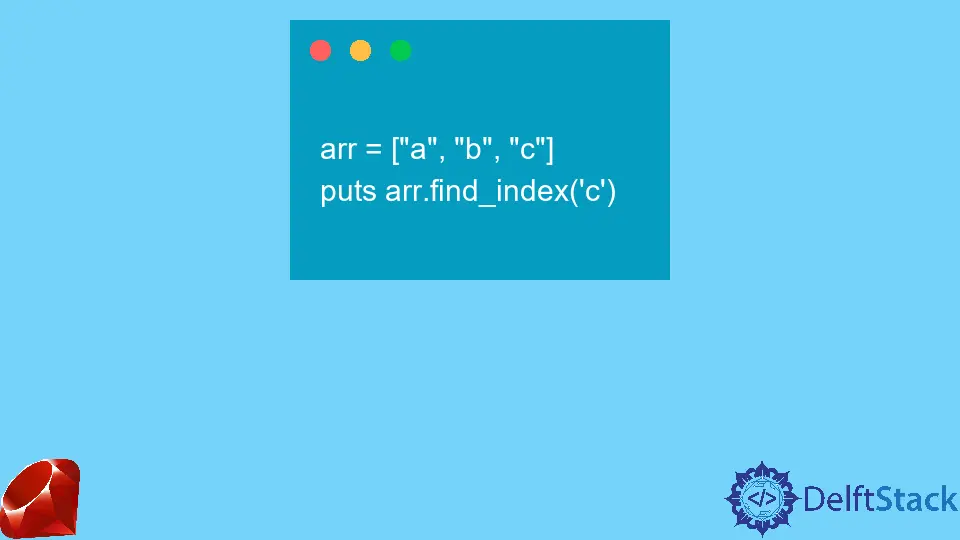
Finding the index of an element in an array is a common task in programming, and Ruby offers several elegant ways to accomplish this. Whether you’re working with a simple list of numbers or a complex array of objects, knowing how to retrieve the index can significantly enhance your coding efficiency.
In this tutorial, we will explore various methods to find an array index in Ruby, complete with practical code examples and detailed explanations. By the end of this article, you’ll have a solid understanding of how to navigate arrays in Ruby and locate elements with ease.
Using the Index Method
One of the simplest ways to find the index of an element in a Ruby array is by using the index
method. This built-in method returns the index of the first occurrence of a specified element. If the element is not found, it returns nil
.
Here’s how you can use it:
array = [10, 20, 30, 40, 50]
index = array.index(30)
puts index
Output:
2
In this example, we define an array containing five integers. We then call the index
method on the array, passing in the value 30
. The method searches through the array and returns the index of the first occurrence of 30
, which is 2
. If we were to search for a value that doesn’t exist in the array, say 60
, the method would return nil
.
This method is particularly useful when you need to find the position of a known element quickly. However, keep in mind that it only returns the index of the first match, so if there are duplicates in the array, you may need to use another method to find all occurrences.
Using the Each_with_index Method
Another powerful way to find the index of elements in a Ruby array is by using the each_with_index
method. This method iterates over the array while also providing the current index, allowing you to perform more complex searches or conditions.
Here’s an example:
array = ['apple', 'banana', 'cherry', 'date']
index = nil
array.each_with_index do |fruit, i|
if fruit == 'cherry'
index = i
break
end
end
puts index
Output:
2
In this code snippet, we have an array of fruit names. We initialize a variable index
to nil
and then use each_with_index
to loop through the array. Inside the block, we check if the current fruit is equal to 'cherry'
. If it is, we assign the current index i
to our index
variable and break out of the loop. The result is that we successfully find the index of 'cherry'
, which is 2
.
Using each_with_index
provides more flexibility than the index
method. You can easily modify the condition to find elements based on various criteria, such as length or starting letter. This makes it a great choice for more complex scenarios.
Using the Find_index Method
Ruby also provides the find_index
method, which is similar to index
, but it allows you to use a block to specify the condition. This means you can define more complex logic to determine which element you want to find.
Here’s how it works:
array = [1, 2, 3, 4, 5]
index = array.find_index { |num| num.even? }
puts index
Output:
1
In this example, we have an array of integers from 1
to 5
. We call find_index
and pass a block that checks if a number is even. The method returns the index of the first even number, which is 1
(corresponding to the number 2
).
This method is particularly useful when you need to find an index based on a condition rather than a specific value. You could easily modify the block to look for numbers greater than 3
, for instance, or any other condition that fits your needs.
Using the Rindex Method
If you need to find the index of the last occurrence of an element in an array, the rindex
method is the way to go. This method works similarly to index
, but it searches from the end of the array towards the beginning.
Here’s an example to illustrate:
array = [1, 2, 3, 2, 1]
index = array.rindex(2)
puts index
Output:
3
In this case, we have an array with duplicate values. When we call rindex
with the value 2
, it returns 3
, which is the index of the last occurrence of 2
in the array.
Using rindex
can be particularly helpful in scenarios where the order of elements matters, and you want to locate the most recent instance of a value. This method is a great addition to your toolkit for working with arrays in Ruby.
Conclusion
Finding the index of an element in an array is a fundamental skill for any Ruby developer. Whether you choose to use the built-in index
, each_with_index
, find_index
, or rindex
methods, each approach has its unique advantages. By understanding these methods and knowing when to apply them, you can enhance your coding efficiency and make your Ruby applications more robust. Now that you have the tools to find array indices, you can confidently tackle a variety of programming challenges.
FAQ
-
What is the difference between index and find_index in Ruby?
index returns the index of the first occurrence of a specific element, while find_index allows you to use a block to specify a condition for finding an index. -
Can I find the index of multiple occurrences in an array?
Yes, you can use methods like each_with_index to loop through the array and collect indices based on your criteria. -
What will rindex return if the element is not found?
If the element is not found, rindex will return nil, similar to the index method. -
Is there a method to find all indices of an element in Ruby?
While Ruby does not have a built-in method for this, you can achieve it using each_with_index and collecting indices in an array. -
Can I use these methods on arrays of strings or objects?
Yes, all these methods work on arrays of any data type, including strings and custom objects, as long as you provide the correct condition or value to search for.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn