How to Check if a File Exists in Ruby
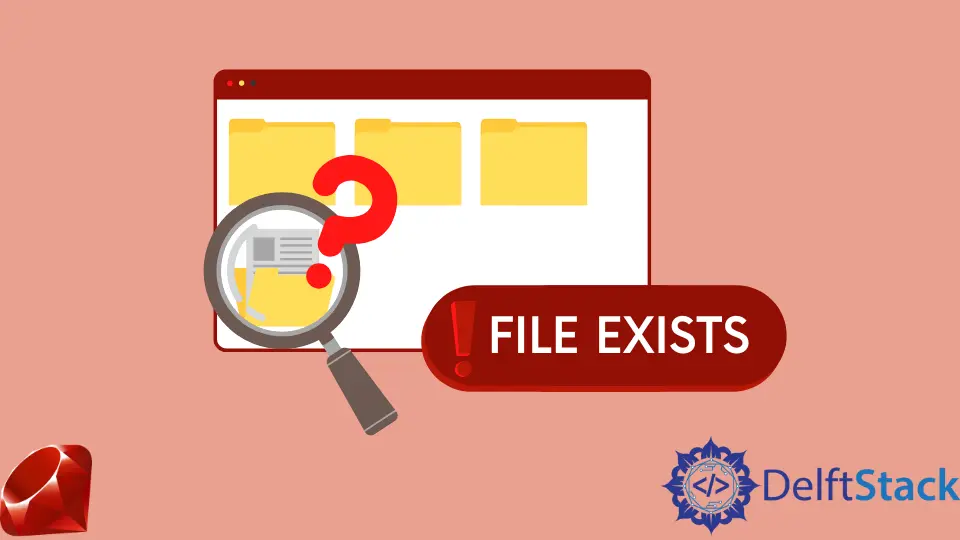
When working with file systems in Ruby, one common task developers encounter is checking if a file exists at a specified path. This task may seem trivial, but it’s crucial for ensuring that your application runs smoothly and handles errors gracefully. Whether you’re building a web application that uploads files, a script that processes data, or a utility that reads configurations, knowing how to verify the existence of a file can save you from potential headaches. In this article, we will explore various methods to check if a file exists in Ruby, complete with code examples and detailed explanations. Let’s dive in!
Using File.exist?
One of the simplest and most straightforward methods to check if a file exists in Ruby is by using the File.exist?
method. This method returns true
if the file exists and false
if it does not. It’s a handy built-in method that makes your code clean and readable.
Here’s how you can use it:
file_path = "example.txt"
if File.exist?(file_path)
puts "The file exists."
else
puts "The file does not exist."
end
Output:
The file does not exist.
In this example, we first define the path of the file we want to check. The File.exist?
method is then called with this path as an argument. If the file exists, it prints a confirmation message; otherwise, it notifies us that the file is missing. This method is particularly useful for quick checks and is easy to implement in any Ruby script.
Using File.file?
Another method to check if a file exists is by using File.file?
. This method not only checks if a file exists but also ensures that the path points to a regular file, not a directory. This makes it a bit more specific than File.exist?
, which returns true for both files and directories.
Here’s an example:
file_path = "example.txt"
if File.file?(file_path)
puts "The file exists and is a regular file."
else
puts "The file does not exist or is not a regular file."
end
Output:
The file does not exist or is not a regular file.
In this code snippet, we check for the existence of a file while ensuring it’s not a directory. The File.file?
method enhances the check by adding an extra layer of validation. This can be particularly useful in applications where distinguishing between files and directories is important.
Using FileTest.exist?
For those who prefer a more modular approach, Ruby provides the FileTest
module, which includes the exist?
method. This method functions similarly to File.exist?
but can be useful in scenarios where you want to keep your file-checking logic organized.
Here’s how it works:
require 'fileutils'
file_path = "example.txt"
if FileTest.exist?(file_path)
puts "The file exists."
else
puts "The file does not exist."
end
Output:
The file does not exist.
In this example, we require the fileutils
library, which is a good practice when working with file operations. The FileTest.exist?
method checks for the file’s existence in the same way as File.exist?
, providing a clean and clear output. Using FileTest
can be particularly beneficial in larger applications where file operations are handled in a modular fashion.
Using begin-rescue
Block
Sometimes, you may want to check if a file exists and handle exceptions that may arise during file operations. In such cases, using a begin-rescue
block can be a robust solution. This method allows you to attempt to open the file and rescue from any errors if it fails.
Here’s an example:
file_path = "example.txt"
begin
File.open(file_path)
puts "The file exists."
rescue Errno::ENOENT
puts "The file does not exist."
end
Output:
The file does not exist.
In this code snippet, we attempt to open the file at the specified path. If the file does not exist, Ruby raises an Errno::ENOENT
exception, which we catch in the rescue
block. This method is particularly useful when you want to perform additional operations on the file after confirming its existence, as it allows for more complex error handling.
Conclusion
Checking if a file exists in Ruby is a fundamental task that can be accomplished using various methods. From the straightforward File.exist?
to the more specific File.file?
, and even the modular FileTest.exist?
, Ruby provides several options to suit your needs. Additionally, employing a begin-rescue
block allows for more robust error handling, ensuring your application can gracefully manage file-related issues. By mastering these techniques, you’ll enhance your Ruby programming skills and create more reliable applications.
FAQ
-
how can I check if a directory exists in Ruby?
You can use theDir.exist?
method to check if a directory exists. It works similarly toFile.exist?
. -
is there a difference between File.exist? and File.file()?
Yes,File.exist?
checks if a file or directory exists, whileFile.file?
checks specifically for regular files. -
can I check for file existence in a specific directory?
Yes, simply provide the full path to the file, including the directory, when using any of the methods discussed. -
how do I check if a file is readable or writable in Ruby?
You can useFile.readable?(file_path)
andFile.writable?(file_path)
to check the file’s permissions. -
what happens if I check for a file that is a symbolic link?
The methods discussed will return true if the symbolic link points to a valid file; otherwise, they will return false.