How to Get Hash Value in Ruby using the fetch() Method
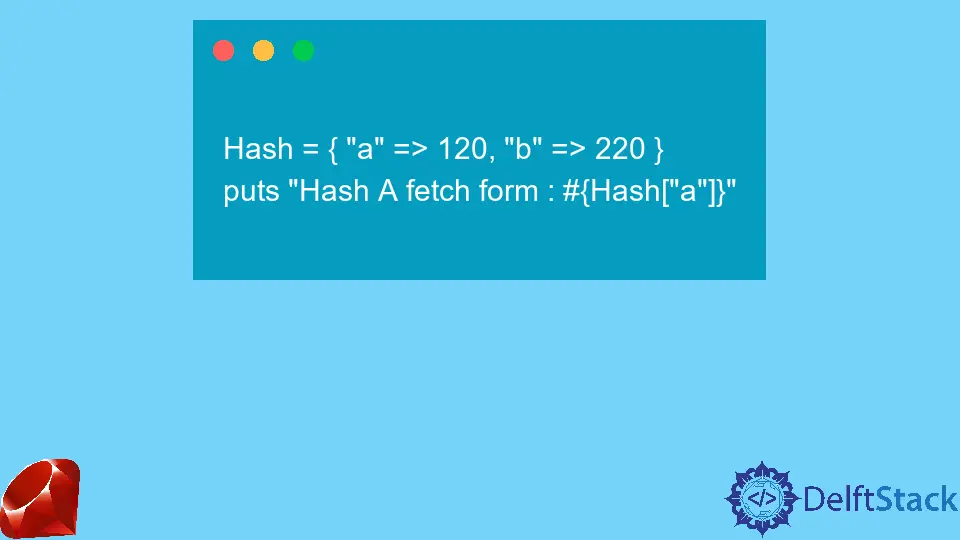
Hash is a very common part of any modern programming language. It is a collection key with corresponding values.
Hash is mostly similar to the array, but the main difference between the Array and Hash is that the index of the array is an integer and generated by the program automatically, but the Hash index the elements by arbitrary keys of any type.
In this article, we are going see different ways to extract data from a Hash and also we are going to see some examples relevant to the topic to make it easier.
Method 1: Get Hash Value Using the fetch()
Method
You can use the method fetch()
to extract data from the Hash. In our example below, we will demonstrate how we can get a value from Hash based on the key using the fetch()
method.
Have a look at the below example.
Hash = { 'a' => 120, 'b' => 220 }
puts "Hash A fetch form : #{Hash.fetch('a')}"
We extract the data with the key A
in the example above. The program above will provide you with the below output.
Hash A fetch form : 120
Method 2: Get Hash Value Using the General Method
You can follow this example if you are not willing to use the fetch()
method. In our example below, we will see how we can extract the data of a specific key from a Hash.
Let’s take a look at the below example.
Hash = { 'a' => 120, 'b' => 220 }
puts "Hash A fetch form : #{Hash['a']}"
We extract the data with the key A
in the example above. The program above will provide you with the below output.
Hash A fetch form : 120
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn