extend in Ruby
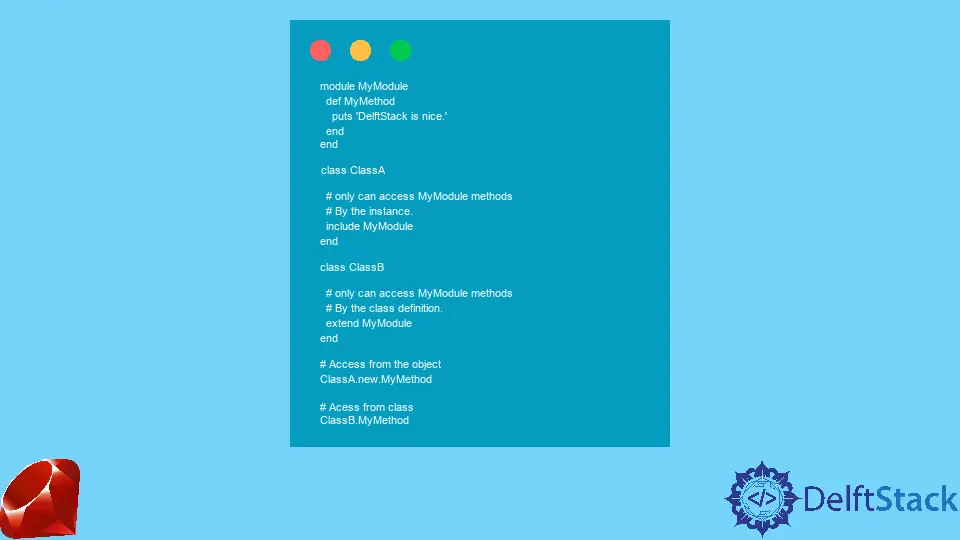
Referencing from class to class and function to function is a common task in Object Oriented Programming. If you are looking for a way to import modules from different classes, then this article can help you.
In Ruby, you can do this in two different ways. By using either include
or extend
.
In this article, we will discuss the include
and extend
in Ruby and look at a relevant example to make the topic easier.
What Is include
in Ruby
include
allows you to import a code module. If you try to get a method of importing the module through the class directly, then you will receive an error as it is imported as a subclass for the superclass.
The solution here is to get it by the instance of the class. You can use include
by using the keyword include
.
What Is extend
in Ruby
Another way to import a module is using the keyword extend
. Through the extend
, you can import a method as a class method.
But if you try to get a method of an imported module through the instance, you will get an error. You must get that method by the class definition to avoid this error.
Use include
and extend
in Ruby
In our example below, we will illustrate the use of include
and extend
keywords in Ruby. Have a look at the below example code.
module MyModule
def MyMethod
puts 'DelftStack is nice.'
end
end
class ClassA
# only can access MyModule methods
# By the instance.
include MyModule
end
class ClassB
# only can access MyModule methods
# By the class definition.
extend MyModule
end
# Access from the object
ClassA.new.MyMethod
# Acess from class
ClassB.MyMethod
Each line’s purpose is commented-out and discussed the working mechanism of the keywords include
and extend
. When you execute the above example code, you will get the below output.
DelftStack is nice.
DelftStack is nice.
Now, if you try to access the method directly from the class like below:
ClassA.MyMethod
You will receive the below error:
# NoMethodError: undefined method
# `MyMethod' for ClassA:Class
This is because the ClassA
includes the module using the keyword include
and include
doesn’t allow you to call a method directly from the class.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn