Difference Between Each_with_index and each.with_index in Ruby
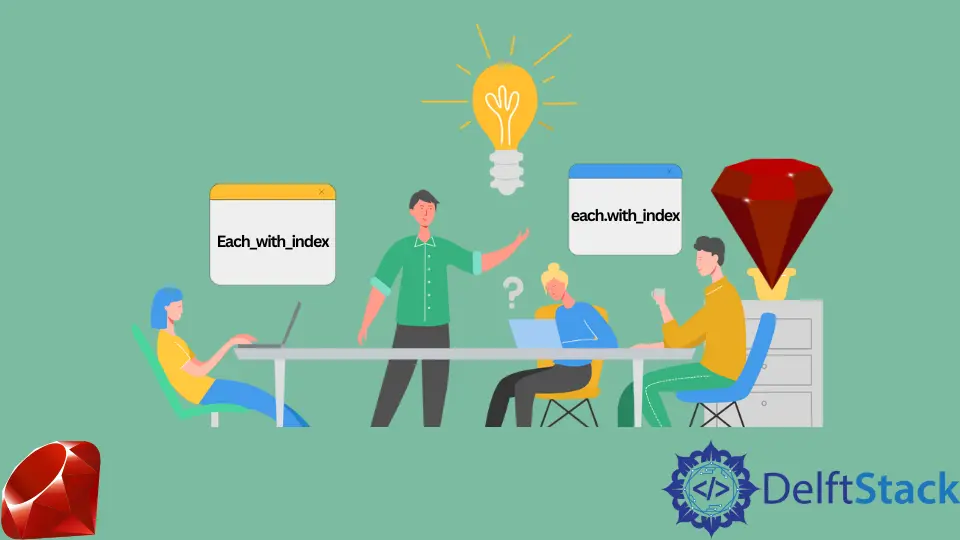
This tutorial will demonstrate the usage of the method each_with_index
and its difference from each.with_index
.
the each_with_index
Method in Ruby
We may occasionally need to iterate through an array and know the current array element index.
The following are examples of naive Ruby programmers:
array = (0..9).to_a
index = 0
array.each do |_num|
# do something
index += 1
end
This code works, but it is not very nice. The first issue is that it added two additional lines of code, and more code means more potential bugs.
The second issue is that the index
variable becomes orphaned after execution. If another chunk of code below uses the same variable name, this could become a problem.
To get around this, Ruby has a method called each_with_index
that allows us to access the current element index while iterating.
array = ('a'..'e').to_a
array.each_with_index do |char, index|
puts "#{index}: #{char}"
end
Output
0: a
1: b
2: c
3: d
4: e
each_with_index
is more elegant than using an extra variable to keep track of the current index. It exposes the index of iteration as the second argument of a block.
As a result, we don’t have to manually increase the index or leave an orphan variable that could cause a severe side effect.
the each.with_index
Method in Ruby
Ruby each.with_index
comes with convenience. It accepts a value that allows the index to start from a number other than 0.
The code below uses the each_with_index
method.
[1,2,3].each_with_index { |n, index| puts "index: #{index}" }
index: 0
index: 1
index: 2
=> [1, 2, 3]
We can write an equivalent code below using the each.with_index
method.
[1,2,3].each.with_index(0) { |n, index| puts "index: #{index}" }
index: 0
index: 1
index: 2
=> [1, 2, 3]
To start the index from 5, using each.with_index
we have,
[1,2,3].each.with_index(5) { |n, index| puts "index: #{index}" }
index: 5
index: 6
index: 7
=> [1, 2, 3]