each and collect Keywords in Ruby
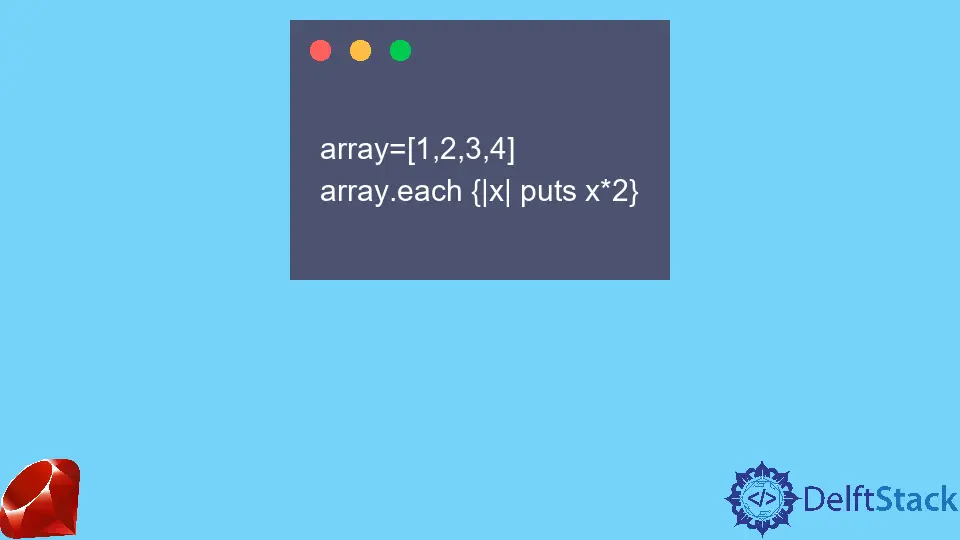
You may be looking for the shortest way to operate on each element of an array. You can design a for
loop, go through all the array elements one by one, and perform the action.
But there is the easiest way available in Ruby. You can use either each
or collect
for this purpose.
In this article, we will discuss the use of each
and collect
in Ruby. Also, we will see some examples relevant to the topic to make it easier.
each
and collect
are two built-in keywords in Ruby that allow you to perform an action on each array element.
Use each
in the Array in Ruby
In our example below, we will see the use of each
in an array. Let’s take the below example from our discussion.
array = [1, 2, 3, 4]
array.each { |x| puts x * 2 }
In the above example, we multiplied each array element by 2 and showed the result. We used each
for this purpose.
After running the above example code, you will get the below output.
2
4
6
8
Use collect
in the Array in Ruby
In our example below, we will demonstrate the use of collect
in an array. Let’s take the below example in our discussion.
array = [1, 2, 3, 4]
puts(array.collect { |x| x * 2 })
In the above example, we multiplied each array element by 2 and showed the result. We used collect
for this purpose.
After running the above example code, you will get the below output.
2
4
6
8
Though the collect
and each
work similarly, they have their own differences. The major difference between the each
and collect
is each
loops over the items and performs actions, but it doesn’t affect the array or create a new object where the collect
applies a specific block of code to all the items and return a new version of the array.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn