Literal for Double-Quoted Strings in Ruby
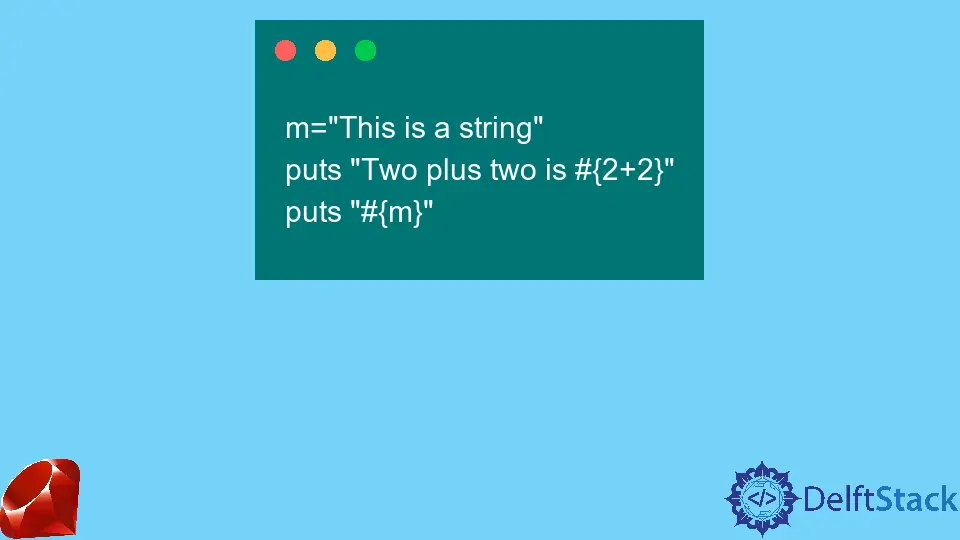
If you are creating a program, you may need to put the value of a variable as an output or want to show the string inside a variable.
In Ruby, we use #{}
for this purpose. The #{}
is used to put the value of a variable in the output text.
In this article, we will see the use of #{}
in Ruby, and also we will look at an example relevant to the topic to make the topic easier.
an Example on #{}
in Ruby
In our example below, we will illustrate how we can use the #{}
in Ruby. Have a look at the below example code.
m = 'This is a string'
puts "Two plus two is #{2 + 2}"
puts "#{m}"
In the example above, we first performed a simple calculation inside the #{}
and attached the result with the output.
puts "Two plus two is #{2 + 2}"
After that, we printed the value of variable m
through the line below.
puts "#{m}"
Now the whole program will show you the below output.
Two plus two is 4
This is a string
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn