The continue Keyword in Ruby
Stewart Nguyen
Feb 09, 2022
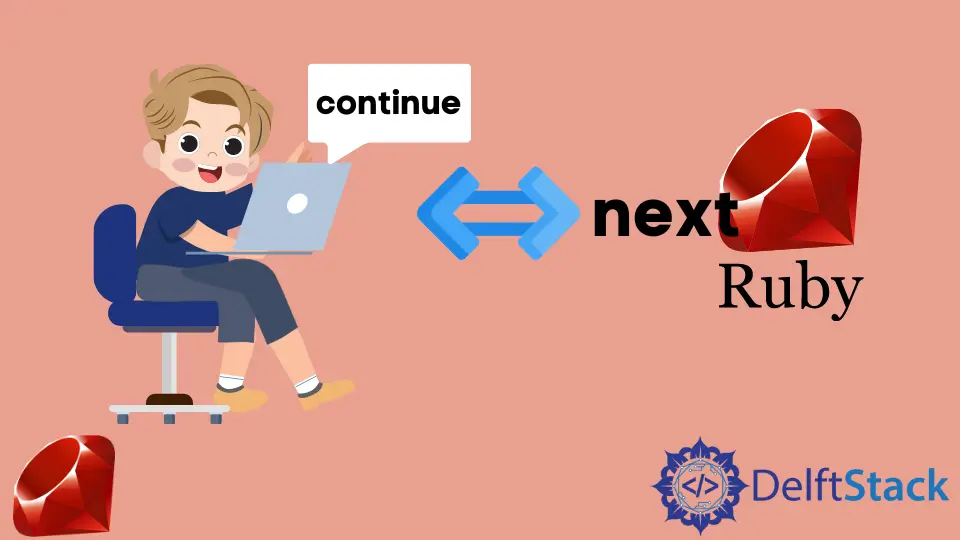
The keyword next
is the ruby equivalent of the continue
keyword in other programming languages. The next
keyword allows you to skip one iteration.
Use the next
Keyword to Skip One Iteration in Ruby Array
Add up all the even numbers in an array using the sum
function. See example:
sum = 0
[1, 2, 3, 4].each do |num|
next if num.odd?
sum += num
end
puts sum
Output:
6
The next
takes an argument and returns nil by default. When used with map
or reduce
, next might produce unexpected results.
We want to add 1
to each even number in an array.
[1, 2, 3, 4].map do |num|
next if num.odd?
num + 1
end
Output:
[nil, 3, nil, 5]
We need to pass next num if num.odd?
to fix it.
[1, 2, 3, 4].map do |num|
next num if num.odd?
num + 1
end
Output:
[1, 3, 3, 5]