Conditional Assignment in Ruby
- What is Conditional Assignment?
- Practical Examples of Using ||=
- Advantages of Using ||= in Ruby
- Conclusion
- FAQ
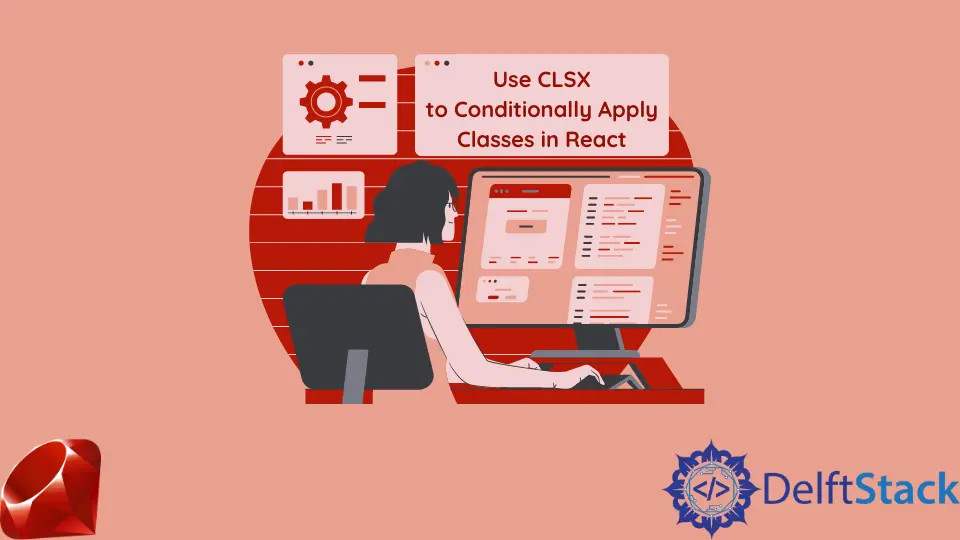
Conditional assignment in Ruby is a powerful feature that allows developers to streamline their code and enhance readability. One of the most commonly used operators for this purpose is ||=
. This operator provides a succinct way to assign a value to a variable only if that variable is currently nil
or false
.
In this article, we will delve into the meaning of ||=
, how it works, and its practical applications in Ruby programming. Whether you are a beginner or an experienced developer, understanding this concept will help you write cleaner and more efficient Ruby code.
What is Conditional Assignment?
Conditional assignment in Ruby allows you to assign a value to a variable based on its current state. The ||=
operator is a shorthand that combines a conditional check and assignment into a single expression. This operator effectively says, “assign this value to the variable only if it’s not already set.”
For example, consider a scenario where you want to set a default value for a variable if it hasn’t been assigned yet. Instead of writing a lengthy conditional statement, you can use ||=
to achieve this with elegance and efficiency.
Here’s a simple example to illustrate this concept:
my_variable ||= "Default Value"
In this line of code, if my_variable
is nil
or false
, it will be assigned the string "Default Value"
. If my_variable
already holds a value, it remains unchanged. This simple yet effective operator helps to reduce boilerplate code and makes your intentions clear at a glance.
Output:
If my_variable is nil, it becomes "Default Value". If not, it retains its original value.
Practical Examples of Using ||=
Now that we understand the basic functionality of the ||=
operator, let’s explore some practical examples that demonstrate its utility in real-world Ruby applications.
Example 1: Setting Default Configuration
In many applications, you might have configuration settings that need default values. Using ||=
, you can easily assign these defaults without cluttering your code.
config = {}
config[:timeout] ||= 30
config[:retry_attempts] ||= 5
In this example, if config[:timeout]
is not already set (i.e., it is nil
), it will be assigned the value 30
. Similarly, if config[:retry_attempts]
is not defined, it will default to 5
. This approach not only simplifies your code but also ensures that your application has sensible defaults.
Output:
config[:timeout] is now 30 if it was nil. config[:retry_attempts] is now 5 if it was nil.
Example 2: Memoization in Methods
Another common use case for ||=
is in the context of memoization. Memoization is a technique where you cache the result of expensive method calls and return the cached result when the same inputs occur again.
def expensive_calculation
@result ||= perform_heavy_computation
end
def perform_heavy_computation
# Simulating a time-consuming operation
sleep(2)
42
end
In this example, the method expensive_calculation
calls perform_heavy_computation
, but only the first time it’s called. The result is stored in the instance variable @result
. On subsequent calls, @result
will already have the computed value, and the heavy computation will be skipped.
Output:
The first call to expensive_calculation takes 2 seconds. Subsequent calls return 42 immediately.
Advantages of Using ||= in Ruby
The ||=
operator brings several advantages to Ruby programming. First and foremost, it enhances code readability. When you see variable ||= value
, it’s immediately clear that you’re dealing with a conditional assignment. This clarity can significantly improve the maintainability of your code.
Additionally, using ||=
reduces the amount of boilerplate code. Rather than writing multiple lines to check if a variable is nil
or false
before assigning a value, you can achieve the same result in a single line. This not only saves time but also minimizes the likelihood of introducing bugs.
Moreover, ||=
is particularly useful in scenarios where default values are essential, such as configuration settings or caching results. By leveraging this operator, you can ensure that your application behaves predictably, even in cases where certain values may not be explicitly set.
Output:
Using ||= improves readability and reduces boilerplate code, making your Ruby applications cleaner and more efficient.
Conclusion
Conditional assignment with the ||=
operator is a powerful feature in Ruby that simplifies code and enhances readability. By allowing you to assign values only when necessary, it helps you write cleaner, more efficient code. Whether you’re setting default values or implementing memoization, understanding how to use ||=
effectively can greatly improve your Ruby programming skills. As you continue to explore Ruby, keep this operator in mind to make your code more elegant and maintainable.
FAQ
-
What does the ||= operator do in Ruby?
The ||= operator assigns a value to a variable only if that variable is currently nil or false. -
Can I use ||= with any data type in Ruby?
Yes, ||= can be used with any data type, including strings, integers, arrays, and hashes. -
Is ||= the same as using if statements for assignment?
While both achieve similar results, ||= is a shorthand that simplifies the code and improves readability. -
Are there any performance implications when using ||=?
Using ||= can improve performance in scenarios like memoization, as it avoids unnecessary computations. -
Can ||= be used in method definitions?
Yes, ||= can be used within methods to set default values for instance variables.