How to Combined Comparison Operator <=> in Ruby
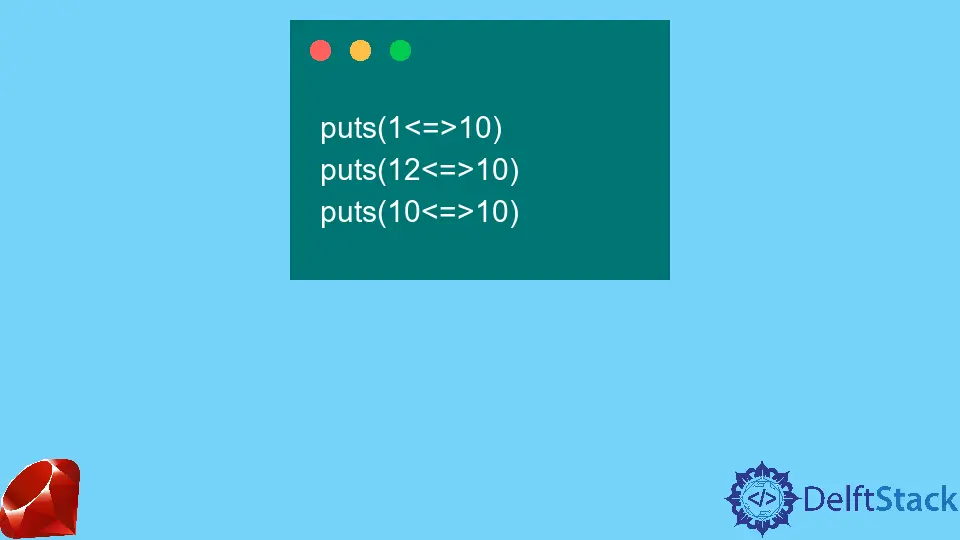
We will introduce the combined comparison operator <=>
in Ruby with an example.
Combined Comparison Operator <=>
in Ruby
We use operators in almost all of our functions. Operators are considered the backbone of any program.
Even from simple functions to complex functions, operators are used everywhere.
Operators can be used to count complex algorithms or create security encryptions. This tutorial will discuss the <=>
operator.
It is known as a combined comparison operator. As we all know, some operators are used to check if the two operands are equal or not.
Some operators tell us whether one operand is greater than the other or not.
But with the help of a combined comparison operator, we can determine all three things without using multiple operators and creating multiple loops for just checking.
We can use this operator to return 0
if the first operand is equal to the second one. It will return 1
if the first operand is greater than the other.
It will return -1
if the first operand is smaller than the other one.
This operand is very useful if we want to create a function to check two operands. Let’s go through an example and create a function that will take in two arguments and check whether both are equal or which one is greater than the other.
puts(1 <=> 10)
puts(12 <=> 10)
puts(10 <=> 10)
Output:
As shown from the above example, when the provided first argument is smaller than the second one, it returns -1
.
When we provided the first argument greater than the second argument, it returned 1
. And when we provided the first and second arguments as equal, it returned 0
.