The Attr_accessor, Attr_reader, and Attr_writer in Ruby
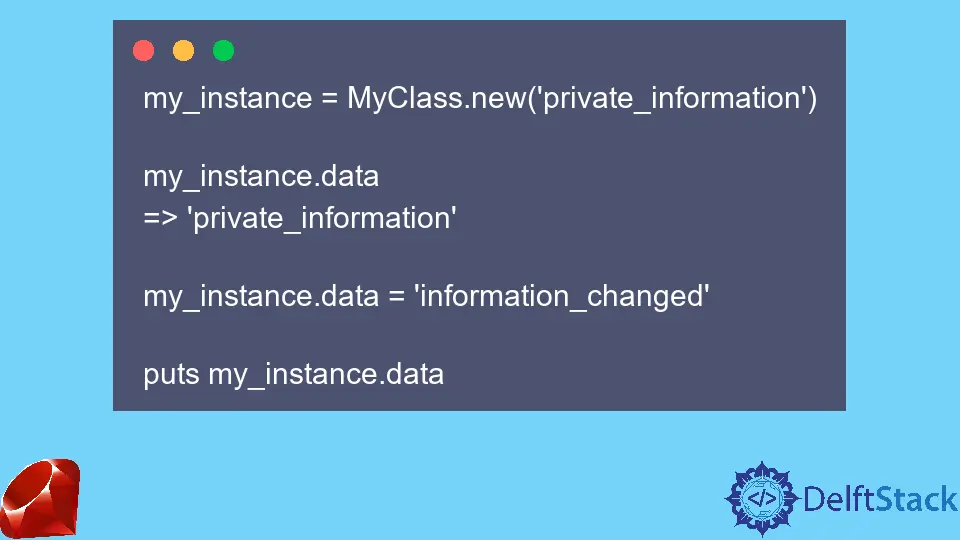
In the world of Ruby programming, understanding how to manage object attributes is crucial for building robust applications. This is where attr_accessor
, attr_reader
, and attr_writer
come into play. These methods are essential for encapsulating and exposing class data, allowing you to control how your objects interact with the outside world. Whether you’re a beginner or an experienced developer, grasping these concepts can significantly enhance your coding efficiency and maintainability.
In this article, we will delve into each of these methods, providing clear examples and explanations to help you understand their unique roles in Ruby’s object-oriented programming paradigm.
Understanding attr_reader
The attr_reader
method is a way to create read-only attributes in your Ruby classes. By using attr_reader
, you can expose instance variables to the outside world without allowing them to be modified directly. This is particularly useful when you want to ensure that the integrity of your object’s state is maintained.
Here’s how you can implement attr_reader
:
class User
attr_reader :name
def initialize(name)
@name = name
end
end
user = User.new("Alice")
puts user.name
Output:
Alice
In this example, we define a class called User
with an instance variable @name
. The attr_reader
method creates a getter method for @name
, allowing external code to read the value of this instance variable. However, since there is no corresponding setter method, the name cannot be changed once it’s set during initialization. This ensures that the user’s name remains constant throughout the object’s lifecycle, which can be crucial for maintaining data integrity.
Exploring attr_writer
On the other hand, attr_writer
allows you to create write-only attributes. This method enables you to set the value of an instance variable from outside the class while preventing direct access to it. This can be particularly useful when you want to control how an attribute is modified.
Let’s see attr_writer
in action:
class User
attr_writer :age
def initialize(name)
@name = name
@age = 0
end
end
user = User.new("Alice")
user.age = 30
Output:
(No output is produced, but the age has been set to 30)
In this case, we define the User
class with an attr_writer
for the @age
instance variable. This allows external code to set the user’s age, but it does not provide a way to read it. This is useful in scenarios where you want to restrict access to sensitive data or simply want to enforce certain conditions on how an attribute is set. For example, you might want to implement validation logic within the setter method to ensure that the age is a positive number.
Utilizing attr_accessor
Finally, attr_accessor
is a combination of both attr_reader
and attr_writer
. It allows you to read and write an instance variable from outside the class. This is the most flexible option when you want to expose an attribute fully.
Here’s how you can use attr_accessor
:
class User
attr_accessor :email
def initialize(name, email)
@name = name
@email = email
end
end
user = User.new("Alice", "alice@example.com")
puts user.email
user.email = "alice.new@example.com"
puts user.email
Output:
alice@example.com
alice.new@example.com
In this example, we define the User
class with attr_accessor
for the @email
instance variable. This allows external code to both read the email and modify it as needed. When we create a new user and set their email, we can easily access and update the email address. This flexibility is particularly useful in applications where user data may change frequently, such as in web applications or APIs.
Conclusion
In summary, attr_reader
, attr_writer
, and attr_accessor
are powerful tools in Ruby that allow you to manage class data effectively. Each method serves a unique purpose, whether you want to restrict access to instance variables or provide full read-and-write capabilities. By utilizing these methods, you can enhance your Ruby classes, making them more maintainable and easier to work with. As you continue your journey in Ruby programming, mastering these concepts will undoubtedly improve your coding skills and help you build better applications.
FAQ
-
What is the difference between attr_reader and attr_writer?
attr_reader creates a getter method for an instance variable, while attr_writer creates a setter method. -
Can I use attr_accessor without initializing an instance variable?
Yes, you can use attr_accessor without initializing an instance variable, but it may lead to nil values if the variable is not set. -
Is it possible to have custom logic in attr_reader or attr_writer?
Yes, you can define custom getter and setter methods if you need to implement additional logic when accessing or modifying an instance variable. -
Can attr_accessor be used for class variables?
No, attr_accessor is designed for instance variables. Class variables require different methods for access. -
When should I use attr_writer instead of attr_accessor?
Use attr_writer when you want to allow writing to an instance variable but not reading it, enforcing encapsulation.