Meaning of Ruby Question Mark ?
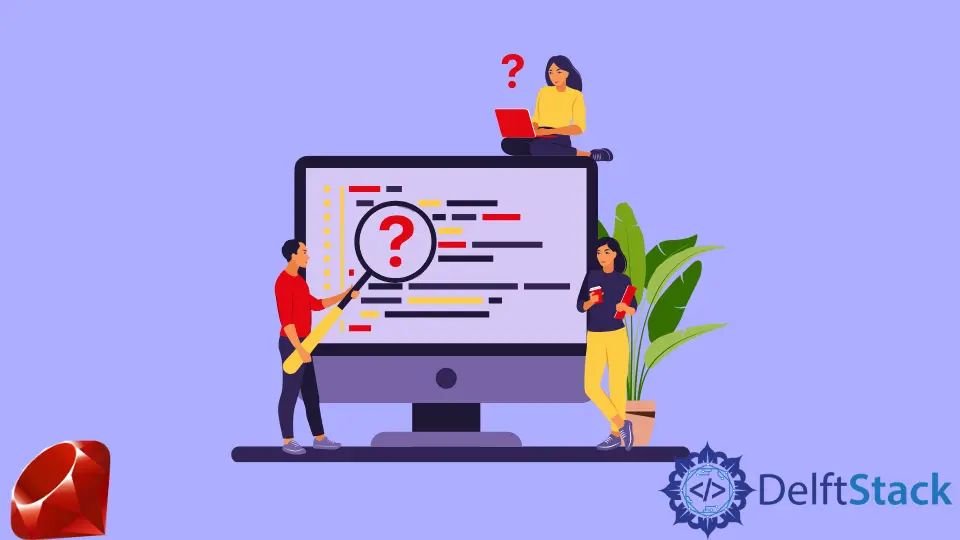
In the world of programming, every language has its quirks and unique features. Ruby, known for its elegant syntax, has a fascinating element that often piques the interest of developers: the question mark ?
. This seemingly simple character plays a significant role in Ruby, particularly when it comes to predicate methods and the ternary operator. Understanding these concepts can enhance your coding skills and improve your ability to write clean, efficient code.
In this article, we will explore what predicate methods are, how the ternary operator works, and provide practical examples to illustrate these concepts. By the end, you’ll have a solid grasp of the meaning and application of the Ruby question mark.
Understanding Predicate Methods in Ruby
In Ruby, a predicate method is a method that returns a boolean value: either true
or false
. These methods are typically used to check the state or condition of an object. The convention in Ruby is to end the method name with a question mark, which helps indicate its purpose at a glance.
Here’s a simple example of a predicate method:
class User
attr_accessor :age
def initialize(age)
@age = age
end
def adult?
@age >= 18
end
end
user = User.new(20)
puts user.adult?
Output:
true
In this code snippet, we define a User
class with an age
attribute. The adult?
method checks if the user’s age is 18 or older. When we create a new user with an age of 20 and call the adult?
method, it returns true
, indicating that the user is indeed an adult.
Using predicate methods can make your code more readable and expressive. By naming methods with a question mark, you signal to other developers that they should expect a boolean result. This practice aligns with Ruby’s philosophy of making code intuitive and self-documenting.
The Ternary Operator in Ruby
The ternary operator is a compact way to express conditional logic in Ruby. It allows you to evaluate a condition and return one of two values based on whether the condition is true or false. The syntax is straightforward: condition ? value_if_true : value_if_false
. This operator is particularly useful for simplifying code and reducing the need for lengthy if-else statements.
Let’s look at an example of the ternary operator in action:
age = 16
status = age >= 18 ? "Adult" : "Minor"
puts status
Output:
Minor
In this example, we have an age
variable set to 16. The ternary operator checks if the age is 18 or older. Since it is not, the expression evaluates to “Minor”. This concise way of handling conditions can make your code cleaner and easier to read, especially when dealing with simple conditions.
However, while the ternary operator is powerful, it’s essential to use it judiciously. Overusing it or nesting multiple ternary operators can lead to code that is difficult to read and maintain. Striking a balance between clarity and brevity is key in Ruby programming.
Conclusion
The question mark ?
in Ruby is more than just a punctuation mark; it signifies critical programming concepts that enhance the language’s readability and efficiency. Predicate methods provide a clear way to check conditions, while the ternary operator allows for concise conditional statements. By mastering these features, you can write cleaner, more expressive Ruby code. Whether you are a beginner or an experienced developer, understanding the meaning of the Ruby question mark can significantly improve your programming skills.
FAQ
-
What are predicate methods in Ruby?
Predicate methods are methods that return a boolean value, typically ending with a question mark to indicate their purpose. -
How does the ternary operator work in Ruby?
The ternary operator evaluates a condition and returns one of two values based on whether the condition is true or false, using the syntaxcondition ? value_if_true : value_if_false
. -
Can I create my own predicate methods?
Yes, you can create your own predicate methods by defining methods that return boolean values and following the naming convention of ending with a question mark. -
Is using the ternary operator recommended for complex conditions?
While the ternary operator is useful for simple conditions, it is generally not recommended for complex logic, as it can reduce code readability. -
What is the advantage of using predicate methods?
Predicate methods improve code readability by clearly indicating that a method checks a condition and returns a boolean value.