Difference Between puts and print in Ruby
Nurudeen Ibrahim
Apr 28, 2022
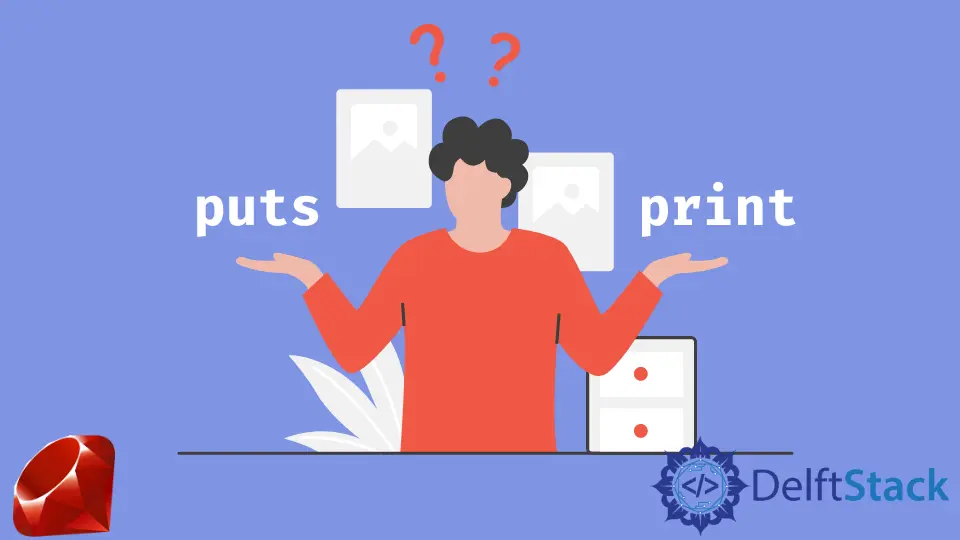
These two methods, puts
and print
, can be used interchangeably in most cases without noticing any difference in the results. But, in some cases where multiple values need to be output, the difference is visible.
Difference Between puts
and print
in Ruby
Basically, puts
adds a new line to the end of each value of its argument but print
does not. Let’s check an example with an array to see the difference.
Example Code Using puts
in Ruby
arr = [1, 2, 3, 6, 7]
puts arr
Output:
1
2
3
6
7
Example Code Using print
in Ruby
arr = [1, 2, 3, 6, 7]
print arr
Output:
[1, 2, 3, 6, 7]
No visible difference is seen for simple arguments such as strings or numbers.
Example Code Using puts
and print
in Ruby
str = 'John'
puts str
print str
Output:
John
John