How to Parse a JSON String in Ruby
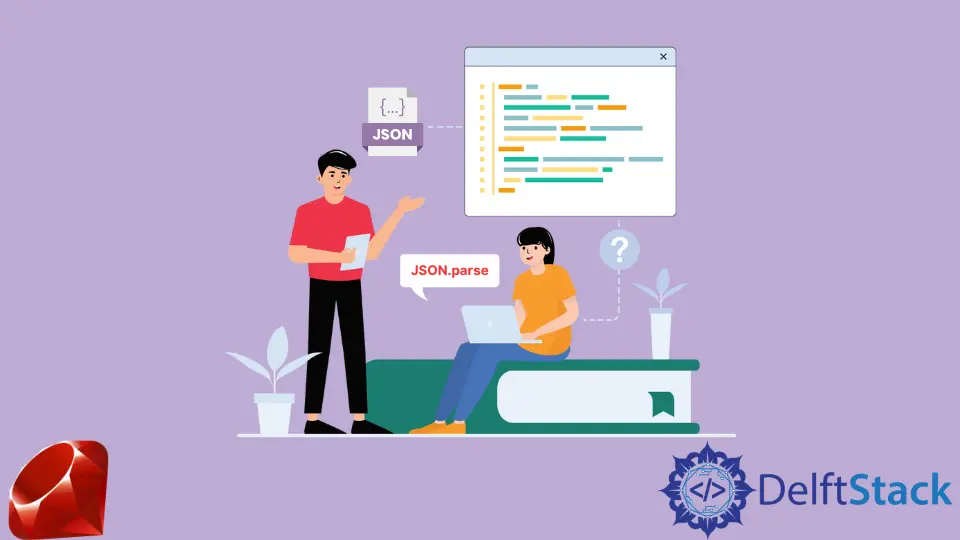
The JSON(JavaScript Object Notation) is a popular format for data interchange, used mostly between web applications to send and receive data. In this tutorial, we will be looking at how to parse JSON strings.
Common examples of JSON value could be:
- a list of values enclosed by square brackets. E.g
["Orange", "Apple", [1, 2]]
- a list of key/pairs enclosed by curly braces. E.g
{ "name": "John", "sex": "male", "grades": [60, 70, 80, 90] }
These JSON values sometimes come in string forms, for example, as a response from an external API, and there’s always a need to convert them into what Ruby can work. There’s a parse
method of the JSON
module that allows us to do that.
Example Codes:
require 'json'
json_string = '["Orange", "Apple", [1, 2]]'
another_json_string = '{ "name": "John", "sex": "male", "grades": [60, 70, 80, 90] }'
puts JSON.parse(json_string)
puts JSON.parse(another_json_string)
Output:
["Orange", "Apple", [1, 2]]
{"name"=>"John", "sex"=>"male", "grades"=>[60, 70, 80, 90]}
To make the JSON module available in our code, we first need to require the file, and that’s what the first line of our code does.
As shown in the example above, JSON.parse
converts the json_string
into a Ruby Array and converts the another_json_string
into a Ruby Hash.
If you have a JSON value that’s not in a string form, you can call to_json
on it to first convert it to a JSON string, then use JSON.parse
to convert it to an Array or Hash as the case may be.
Example Codes:
require 'json'
json_one = ['Orange', 'Apple', [1, 2]]
json_two = { "name": 'John', "sex": 'male', "grades": [60, 70, 80, 90] }
puts JSON.parse(json_one.to_json)
puts JSON.parse(json_two.to_json)
Output:
["Orange", "Apple", [1, 2]]
{"name"=>"John", "sex"=>"male", "grades"=>[60, 70, 80, 90]}