The or Operator in Ruby
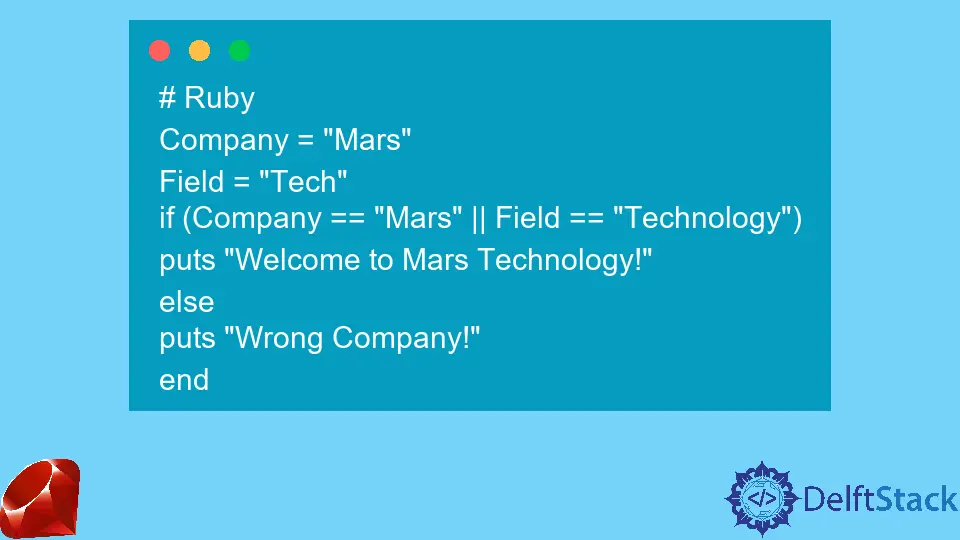
We will introduce how to use or operator
in Ruby.
the or
Operator (||
) in Ruby
There are many situations where we need to implement certain tasks based on two conditions. If these situations are true, we use logical operators if we want a certain task to run automatically.
Operators are used to performing different tasks based on conditions. We can use the or
operator (||
) for this purpose.
The or
operator (||
) works so that we pass two conditions. The task will be performed if any of the conditions return true
.
We use the following sign ||
to perform or
logical operator in Ruby. Let’s go through an example where we will use this operator to perform a certain task.
Example Code:
# Ruby
Company = 'Mars'
Field = 'Tech'
if Company == 'Mars' || Field == 'Technology'
puts 'Welcome to Mars Technology!'
else
puts 'Wrong Company!'
end
Output:
In the above output, only one condition was true
. It still takes it as true
because the or
operator converts the whole situation to true
if only one of the variables is returning true
.
Let’s have another example in which we will try to use the or
keyword instead of the ||
operator and try to get the result.
Example Code:
# Ruby
Company = 'Mars'
Field = 'Tech'
if Company == 'Mars' or Field == 'Technology'
puts 'Welcome to Mars Technology!'
else
puts 'Wrong Company!'
end
Output:
In the above example, it also returned the same result. It proves that we can use either the or
keyword or ||
operator to get the same logic in Ruby.