How to Get Maximum and Minimum Number in a Ruby Array
Nurudeen Ibrahim
Feb 02, 2024
-
Use the
min
Method to Get Minimum Number in an Array in Ruby -
Use the
max
Method to Get Maximum Number in an Array in Ruby -
Use the
minmax
Method in Ruby
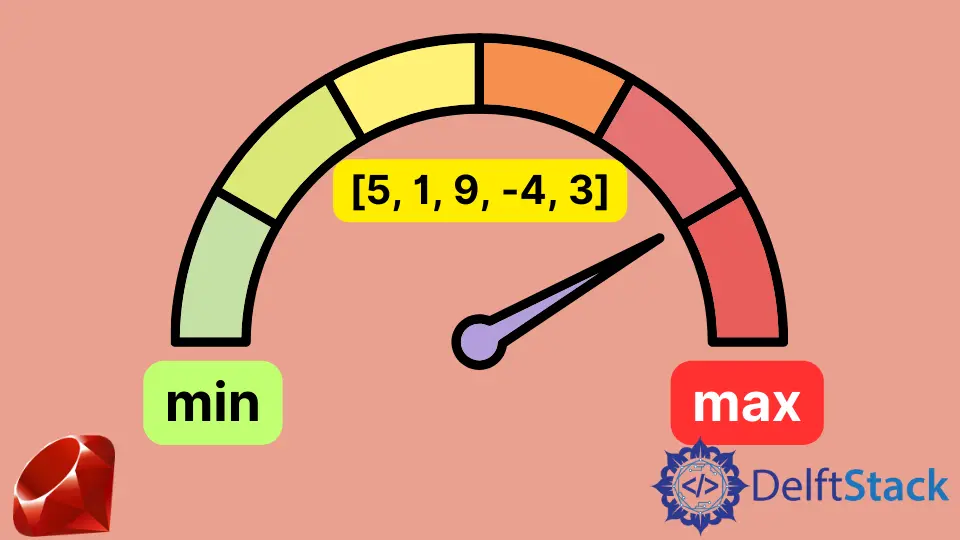
Ruby’s Array
class includes the Enumerable
module and its implementation. As a result, it has access to some Enumerable
methods.
The max
and min
methods are some of the Enumerable
methods used to get the maximum and the minimum number of an Array.
Use the min
Method to Get Minimum Number in an Array in Ruby
Example Code:
numbers = [5, 1, 9, -4, 3]
puts numbers.min
puts numbers.min(2)
Output:
-4
[-4, 1]
Calling min
without an argument returns the minimum number (element
) but returns the n-smallest
numbers if argument "n"
is passed to it.
Use the max
Method to Get Maximum Number in an Array in Ruby
Example Code:
numbers = [5, 1, 9, -4, 3]
puts numbers.max
puts numbers.max(2)
Output:
9
[9, 5]
If max
is called without an argument, it returns the biggest number (element
). If argument "n"
is passed to it, it returns the n-largest
numbers.
Use the minmax
Method in Ruby
The minmax
method returns a two-element array that contains the minimum
and the maximum
value in the given array.
Example Code:
numbers = [5, 1, 9, -4, 3]
puts numbers.minmax
Output:
[-4, 9]