How to Use the Freeze Method in Ruby
- Understanding the Freeze Method
- Freezing Arrays
- Freezing Hashes
- Practical Use Cases for Freeze
- Conclusion
- FAQ
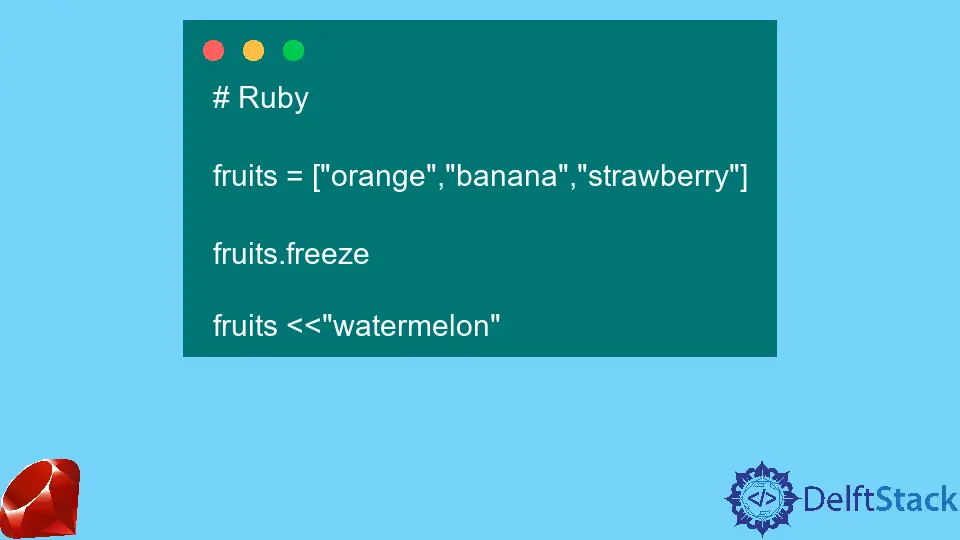
When working with Ruby, you may encounter the freeze
method, a powerful tool that helps you manage object mutability. In Ruby, mutability refers to whether an object can be changed after it has been created. The freeze
method is particularly useful when you want to ensure that an object remains unchanged throughout its lifecycle. By freezing an object, you prevent any modifications, which can be beneficial for maintaining data integrity and avoiding unintended side effects.
This tutorial will walk you through the workings of the freeze
method, providing practical examples and explanations to help you grasp its significance in Ruby programming.
Understanding the Freeze Method
The freeze
method is called on an object to make it immutable. When an object is frozen, any attempt to modify it will raise a FrozenError
. This feature is especially useful in scenarios where you want to create constants or ensure that certain data remains unchanged.
Here’s a simple example to illustrate how the freeze
method works:
str = "Hello, World!"
str.freeze
str << " This will raise an error."
Output:
FrozenError: can't modify frozen String
In this example, we create a string and then freeze it. When we try to modify the string by appending more text, Ruby raises a FrozenError
, indicating that the object cannot be changed.
Freezing objects can help prevent accidental modifications, making your code more robust and reliable. It’s particularly useful when passing objects to methods, ensuring that the original object remains unchanged.
Freezing Arrays
The freeze
method can also be applied to arrays, ensuring that their contents cannot be altered. This is particularly useful when you want to maintain a constant list of items throughout your program.
Consider the following example:
arr = [1, 2, 3]
arr.freeze
arr << 4
Output:
FrozenError: can't modify frozen Array
In this case, we create an array and freeze it. Attempting to append a new element results in a FrozenError
, similar to what we saw with strings. By freezing the array, we ensure that its contents remain constant, which can be crucial in maintaining data integrity, especially in larger applications.
Freezing Hashes
Just like strings and arrays, hashes can also be frozen. Freezing a hash prevents any modifications to its key-value pairs, which can be particularly useful when you want to maintain a constant set of configurations or options.
Here’s an example of freezing a hash:
config = { host: "localhost", port: 3000 }
config.freeze
config[:host] = "127.0.0.1"
Output:
FrozenError: can't modify frozen Hash
In this example, we define a hash with configuration settings and freeze it. When we attempt to change the value associated with the :host
key, Ruby raises a FrozenError
. Freezing hashes can help prevent accidental changes to configurations, making your applications more predictable and easier to debug.
Practical Use Cases for Freeze
Using the freeze
method can significantly enhance your code’s reliability and maintainability. Here are a few practical scenarios where freezing objects can be beneficial:
-
Constants: When defining constants, freezing ensures that their values remain unchanged throughout the program. This is crucial for maintaining consistency.
-
Shared Data: In multi-threaded applications, freezing shared data can help prevent race conditions, where multiple threads try to modify the same object simultaneously.
-
Configuration Settings: By freezing configuration settings, you can prevent accidental changes that may lead to unexpected behavior in your application.
-
Immutable Data Structures: In functional programming paradigms, immutability is a key concept. Using
freeze
allows you to create immutable data structures, leading to cleaner and more maintainable code.
By incorporating the freeze
method into your Ruby programming practices, you can create more robust applications that are less prone to bugs and errors.
Conclusion
The freeze
method in Ruby is a valuable tool for managing object mutability and ensuring data integrity. By making objects immutable, you can prevent unintended modifications and create more reliable and maintainable code. Whether you’re working with strings, arrays, or hashes, understanding how to effectively use the freeze
method can greatly enhance your Ruby programming skills. As you continue to build applications, consider incorporating freezing where appropriate to help safeguard your data and maintain the integrity of your code.
FAQ
-
What happens when I call
freeze
on an object?
When you callfreeze
on an object, it becomes immutable. Any attempt to modify it will raise aFrozenError
. -
Can I unfreeze a frozen object in Ruby?
No, once an object is frozen in Ruby, it cannot be unfrozen. You would need to create a new object if you want to modify the data. -
Is using
freeze
good practice in Ruby?
Yes, usingfreeze
can help maintain data integrity and prevent unintended modifications, making your code more robust. -
Can I freeze nested objects in Ruby?
Yes, you can freeze nested objects, but you need to callfreeze
on each object individually. Freezing a parent object does not automatically freeze its children. -
Are there performance implications of using
freeze
?
While there may be minor performance implications due to immutability checks, the benefits of maintaining data integrity often outweigh these concerns.