for vs each in Ruby
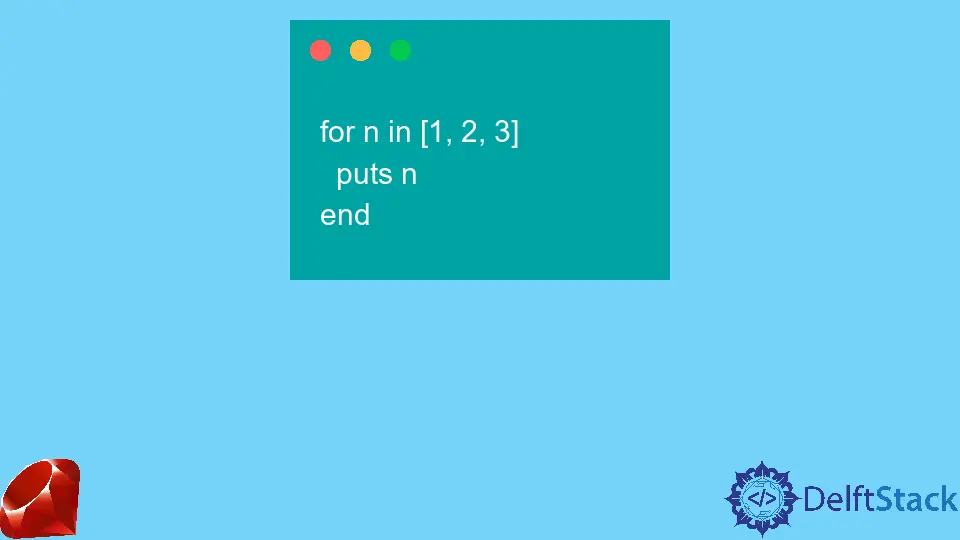
In Ruby, you can iterate through an array using both Array#each
and for..in
. However, there is a significant difference between the two.
Array#each
in Ruby
The Ruby Array#each
method is the most straightforward and widely used method for iterating individual items in an array.
each
is a built-in method of class Array, and it is the most popular way to iterate over the items in Ruby array.
each
takes a block as a parameter and returns the current item.
[1, 2, 3].each { |n| puts n }
Output:
1
2
3
the for..in
Loop in Ruby
Like most other programming languages, Ruby has a for
statement for looping through an array.
for
is followed by a variable as the current element and an iterated array.
for n in [1, 2, 3]
puts n
end
Output:
1
2
3
Under the hood, Array#each
and for..in
almost do the same thing. However, there is one minor difference, which could cause a severe problem.
[1, 2, 3].each { |n| }
puts n
Output:
NameError (undefined local variable or method `n' for main:Object)
Code Sample:
for n in [1, 2, 3]
n
end
puts n
Output:
3
We get an error if we try to print n
after Array#each
, but it prints 3
after the for
loop.
What’s going on?
Before for..in
is executed, the variable n
is defined in advance. After the block is completed, it remains visible outside the block.
In other words, the for
loop is similar to:
n = nil
[1, 2, 3].each { |i| n = i }
puts n
Output:
3
In the case of Array#each
, the variable n
is defined within the block { |n| puts n }
, hence it is not visible to anything outside the scope of the block.
Using a for
loop may cause a frustrating issue by introducing an extra variable as a side effect. As a result, Array#each is prefered over for..in
.