How to Fix Undefined Method in Ruby
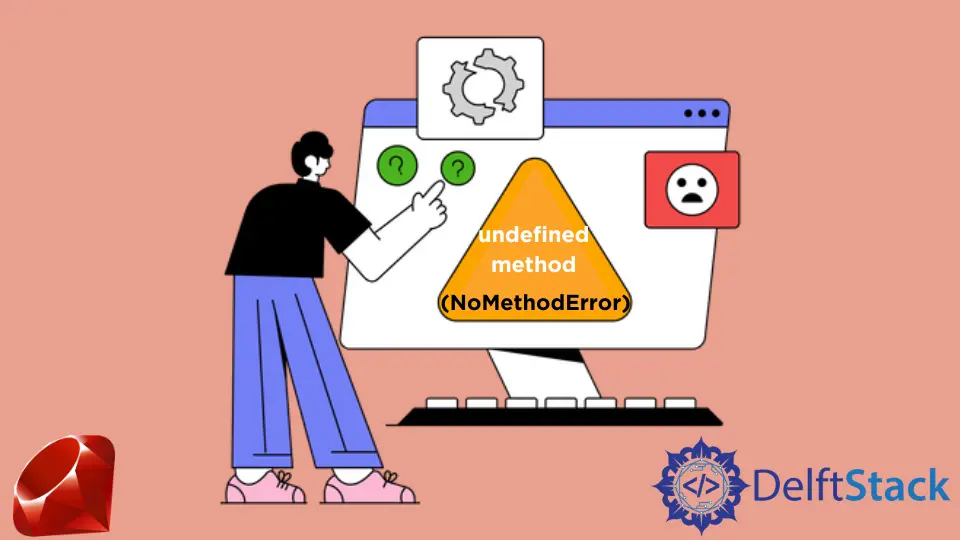
This article will discuss fixing the most common error in Ruby projects, the undefined method.
Fix Undefined Method in Ruby
The undefined method is also called the NoMethodError
exception, and it’s the most common error within projects, according to The 2022 Airbrake Error Data Report.
It occurs when a receiver (an object) receives a method that does not exist.
Undefined Method on an Inbuilt Object
The code below demonstrates how to trigger a NoMethodError
error on an array object if we call an undefined method maximum
on it.
Example code:
arr = [1, 2, 3, 7, 4]
puts arr.maximum
Output:
undefined method `maximum' for [1, 2, 3, 7, 4]:Array (NoMethodError)
Undefined Method on a Custom Object
The code below demonstrates how to trigger a NoMethodError
error on a custom object.
Example code:
class Employee
def initialize(last_name, first_name)
@last_name = last_name
@first_name = first_name
end
def full_name
"#{@first_name} #{@last_name}"
end
end
employee1 = Employee.new('John', 'Doe')
puts employee1.fullname
Output:
undefined method `fullname' for #<Employee:0x0000565026778608> (NoMethodError)
Did you mean? full_name
In the example above, we intentionally used fullname
instead of full_name
, and as a result, Ruby could not find a match but found a name that looked very close. Hence, Did you mean? full_name
was added to the error traceback.