How to Find a Value in a Ruby Array
- Using the include? Method
- Using the index Method
- Using the find Method
- Using the select Method
- Conclusion
- FAQ
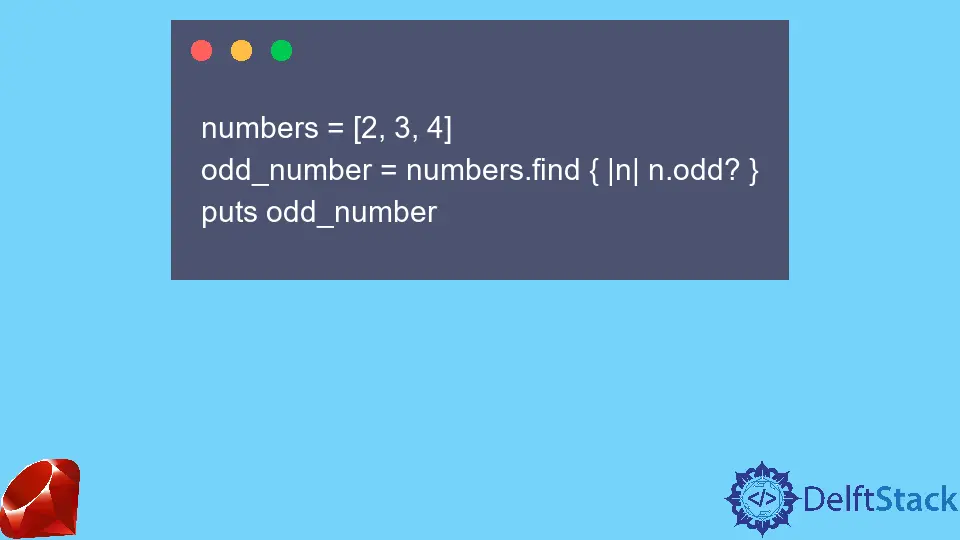
Finding a value in a Ruby array can be essential for various programming tasks, whether you’re developing a simple application or working on complex data manipulations. Ruby arrays are versatile and provide multiple methods to locate values efficiently.
In this tutorial, we’ll explore several effective ways to find a value in a Ruby array, ensuring you have the tools you need to handle this common programming challenge. Whether you’re a beginner or an experienced Ruby developer, understanding how to navigate arrays will enhance your coding skills and improve your applications’ performance. So, let’s dive into the world of Ruby arrays and discover how to find values seamlessly!
Using the include? Method
One of the simplest ways to check if a value exists in a Ruby array is by using the include?
method. This method returns true
if the specified value is present in the array and false
otherwise. It’s straightforward and perfect for quick checks.
fruits = ["apple", "banana", "cherry", "date"]
exists = fruits.include?("banana")
puts exists
Output:
true
In this example, we created an array named fruits
containing several fruit names. By calling fruits.include?("banana")
, we check if “banana” is part of the array. The method returns true
, indicating that the value exists. The include?
method is quick and efficient for small to moderately sized arrays, making it a go-to choice for many developers.
Using the index Method
Another effective way to find a value in a Ruby array is by using the index
method. This method returns the first index of the specified value if it exists, or nil
if it does not. This can be particularly useful when you need to know the position of the value within the array.
numbers = [10, 20, 30, 40, 50]
index = numbers.index(30)
puts index
Output:
2
In this code snippet, we created an array called numbers
with several integer values. By invoking numbers.index(30)
, we find the index of the value 30
. The method returns 2
, which is the position of 30
in the array (keeping in mind that indexing starts at 0
). If the value were not found, index
would return nil
. This method is particularly helpful when you not only want to verify the existence of a value but also need to know where it is located.
Using the find Method
The find
method is another powerful tool for locating a value in a Ruby array. This method iterates through the array and returns the first element that meets the specified condition. If no element matches, it returns nil
. This is particularly useful when you’re dealing with more complex data types or conditions.
products = [{name: "Laptop", price: 999}, {name: "Smartphone", price: 499}, {name: "Tablet", price: 299}]
product = products.find { |item| item[:name] == "Smartphone" }
puts product
Output:
{:name=>"Smartphone", :price=>499}
In this example, we have an array of hashes representing different products. The find
method is used to search for the product with the name “Smartphone”. The block checks each item in the array, and when it finds the match, it returns the entire hash for that product. If no product matched the criteria, it would return nil
. This method is particularly useful for searching through arrays of objects or hashes, allowing for more complex queries.
Using the select Method
If you want to find all occurrences of a value or all elements that meet a certain condition, the select
method is your best friend. Unlike find
, which returns only the first match, select
returns an array of all matching elements.
scores = [85, 92, 75, 92, 88]
high_scores = scores.select { |score| score >= 90 }
puts high_scores
Output:
[92, 92]
In this snippet, we have an array of scores. By using the select
method with a block that checks if each score is greater than or equal to 90
, we retrieve all high scores. The result is a new array containing all scores that meet the condition. This method is particularly useful when you want to filter data based on specific criteria, providing you with a comprehensive view of all matches.
Conclusion
Finding a value in a Ruby array is a fundamental skill every developer should master. From the simple include?
method to the more complex select
method, Ruby provides a rich set of tools for efficiently locating values within arrays. Each method has its unique advantages, depending on your specific needs. Whether you’re checking for existence, retrieving indices, or filtering data, knowing how to navigate Ruby arrays will enhance your programming capabilities and streamline your coding process. So, the next time you need to find a value in a Ruby array, you’ll have the right techniques at your fingertips.
FAQ
-
How do I check if a value exists in a Ruby array?
You can use theinclude?
method to check if a specific value exists in a Ruby array. -
What does the
index
method return in Ruby?
Theindex
method returns the first index of the specified value in the array ornil
if the value is not found. -
Can I find multiple values in a Ruby array?
Yes, you can use theselect
method to find all values that meet a certain condition in a Ruby array. -
Is the
find
method suitable for complex conditions?
Yes, thefind
method is ideal for searching through arrays with more complex conditions by using a block. -
What happens if the value I’m searching for is not in the array?
If the value is not found, methods likeindex
andfind
will returnnil
, whileinclude?
will returnfalse
.