How to Filter an Array in Ruby
-
Using the
select
Method -
Using the
reject
Method -
Using the
filter
Method -
Using the
map
Method with Conditional Logic - Conclusion
- FAQ
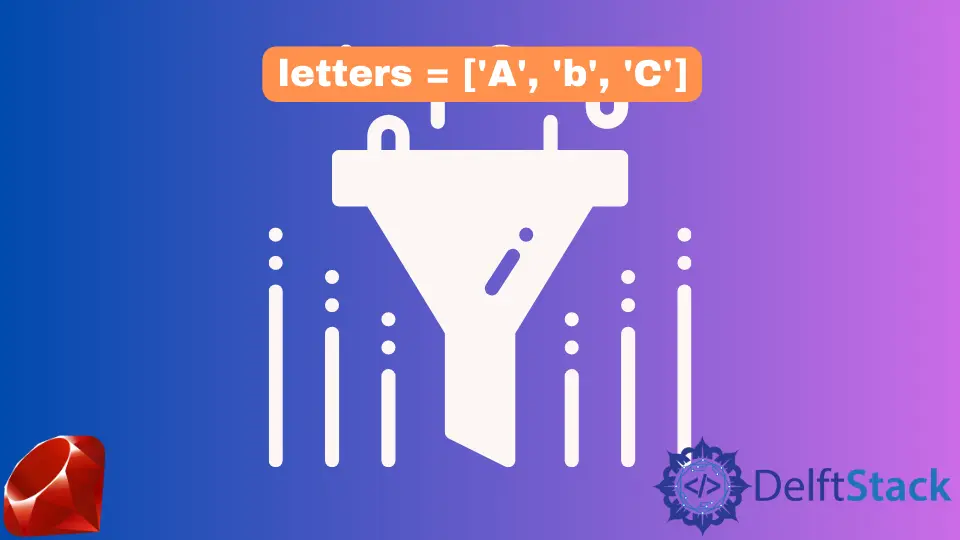
When working with arrays in Ruby, you often need to filter out elements based on specific conditions. Whether you’re looking to extract even numbers, find strings that match a certain pattern, or filter out unwanted data, Ruby offers several intuitive methods to achieve this.
In this article, we’ll explore various techniques to filter arrays effectively, complete with code examples and detailed explanations. By the end, you’ll have a solid understanding of how to manipulate arrays in Ruby to suit your needs. Let’s dive in!
Using the select
Method
One of the most commonly used methods to filter an array in Ruby is the select
method. This method allows you to choose elements that meet a specific condition. The select
method iterates through each element in the array and returns a new array containing all elements for which the block returns true.
Here’s a simple example that filters even numbers from an array:
numbers = [1, 2, 3, 4, 5, 6]
even_numbers = numbers.select { |number| number.even? }
puts even_numbers
Output:
2
4
6
In this example, we start with an array of numbers from 1 to 6. By using the select
method, we pass a block that checks if each number is even. The result is a new array containing only the even numbers: 2, 4, and 6. The beauty of the select
method lies in its readability and straightforwardness, making it a favorite among Ruby developers when filtering arrays.
Using the reject
Method
While the select
method is great for keeping elements that meet certain criteria, the reject
method does the opposite. It creates a new array by excluding elements that match the condition specified in the block. This can be particularly useful when you want to filter out unwanted items.
Here’s an example that filters out odd numbers from an array:
numbers = [1, 2, 3, 4, 5, 6]
odd_numbers_rejected = numbers.reject { |number| number.odd? }
puts odd_numbers_rejected
Output:
2
4
6
In this case, we started with the same array of numbers, but this time we used the reject
method. The block checks if a number is odd, and if it is, that number is excluded from the resulting array. The end result is an array containing only the even numbers again: 2, 4, and 6. The reject
method is particularly handy when you want to filter out specific unwanted elements without needing to create a more complex condition.
Using the filter
Method
In Ruby, filter
is an alias for select
, which means it works in exactly the same way. However, using filter
can enhance code readability by making your intentions clearer. This method returns a new array containing all elements for which the block returns true, just like select
.
Here’s how you can filter strings from an array based on their length:
words = ["apple", "banana", "pear", "kiwi", "grape"]
short_words = words.filter { |word| word.length <= 4 }
puts short_words
Output:
pear
kiwi
In this example, we have an array of fruit names and we want to filter out the words that have four or fewer letters. The filter
method does just that, returning an array with “pear” and “kiwi”. This method is particularly useful when you want to maintain a consistent naming convention in your code, as it conveys the same meaning as select
but may resonate better with your coding style.
Using the map
Method with Conditional Logic
While map
is primarily used for transforming elements of an array, you can also employ it in combination with conditional logic to filter elements. By mapping over the array and using nil
for unwanted elements, you can effectively filter out items.
Here’s an example that filters numbers greater than 3:
numbers = [1, 2, 3, 4, 5, 6]
filtered_numbers = numbers.map { |number| number > 3 ? number : nil }.compact
puts filtered_numbers
Output:
4
5
6
In this case, we use the map
method to iterate over the array. For each number, we check if it’s greater than 3. If it is, we keep the number; otherwise, we return nil
. After mapping, we use the compact
method to remove any nil
values from the resulting array. The final output is an array containing only the numbers greater than 3: 4, 5, and 6. This method is less common for filtering but can be effective in scenarios where transformation is also needed.
Conclusion
Filtering arrays in Ruby is a straightforward process thanks to the various methods available. Whether you choose to use select
, reject
, filter
, or even map
with conditional logic, each method has its unique advantages and can be applied based on your specific needs. Understanding these techniques will not only enhance your Ruby skills but also improve your ability to manipulate data efficiently. Now that you have a solid grasp of how to filter arrays in Ruby, you can confidently apply these methods in your projects.
FAQ
-
What is the difference between
select
andreject
in Ruby?
select
returns an array of elements that meet a condition, whilereject
returns an array of elements that do not meet a condition. -
Can I use
filter
andselect
interchangeably?
Yes,filter
is an alias forselect
, so they function the same way. -
How can I filter an array of strings based on length?
You can use theselect
orfilter
method with a block that checks the length of each string. -
Is it possible to filter arrays without using blocks?
Yes, you can use methods likegrep
to filter arrays based on patterns without explicitly defining a block. -
What is the best method for filtering large arrays in Ruby?
The best method depends on your specific use case, butselect
andreject
are generally efficient and easy to read.