Double Colon :: Operator in Ruby
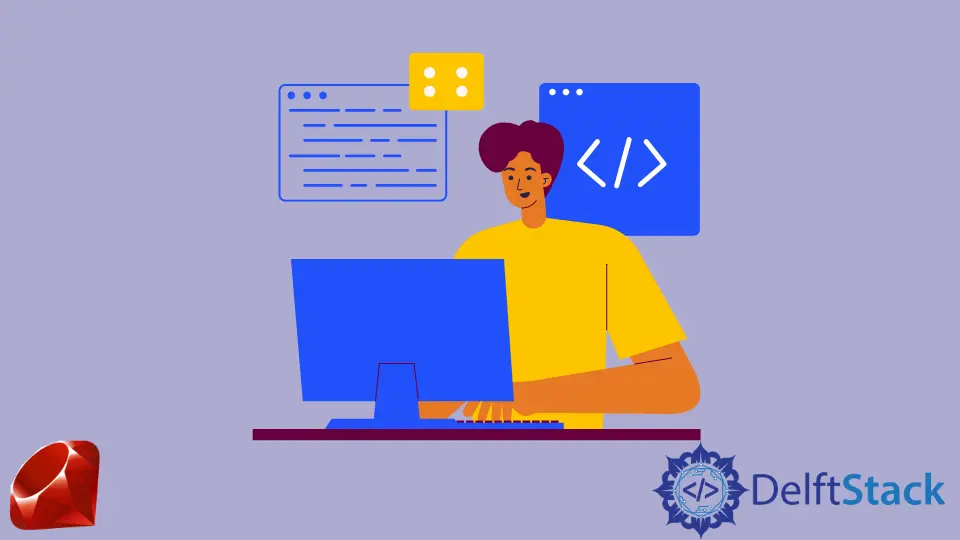
We will introduce how to use the double colon ::
operator in Ruby.
Double Colon ::
Operator in Ruby
Ruby is renowned for its use in automation tools, static websites, and data processing services. We can also use Ruby as a scripting language.
The basic definition of the operator is that they are the symbols representing a specific action. In computer programming languages, many operators, such as Boolean operators, arithmetic operators, and logical operators, are also used in different programming languages.
Operators are the main part of any software built using programming languages. Every software performs some kind of operations using the operators.
Double Colon ::
is a very useful operator that allows constants, classes, or modules to access instance and class methods in Ruby programing language. This operator can access it from anywhere in the module and class.
To start the working of this operator, we first clarify the prefix with ::
constant name with a specific expression that returns a class object. Let’s have an example where we will create a module, Count
.
Inside the count
module, we will create another module named Counter
, that will contain a class CounterClass
containing a constant. We will call this constant using the double colon operators as shown below.
Example Code:
# Ruby
module Count
module Counter
class CounterClass
Ruby_count = 6
end
end
end
puts Count::Counter::CounterClass::Ruby_count
Output:
6
From the above example, we can access any method, class, or constant from any modules using the double colon ::
operators in Ruby.