Difference Between require and require_relative in Ruby
Nurudeen Ibrahim
Apr 28, 2022
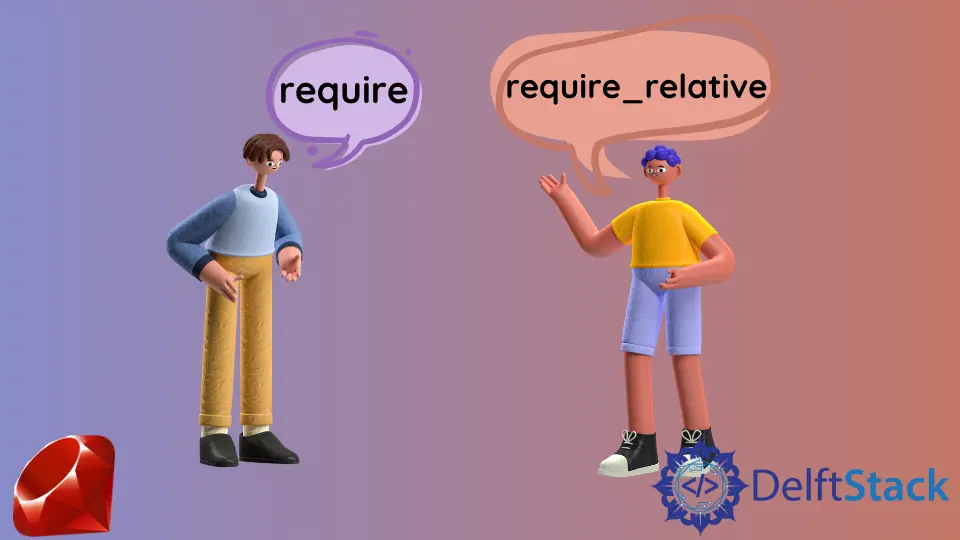
This article will demonstrate the differences between the uses of require and require-relative in Ruby.
Difference Between require
and require_relative
in Ruby
These two methods behave in somewhat similar ways, but with some differences.
Use require_relative
to Import Your Code From Another File
The require_relative
method is used when you need to bring in your code from another file in the same project.
For example, say, we have my-project/lib.rb
and my-project/data.rb
. To import data.rb
to lib.rb
, inside lib.rb
, you can write:
require_relative 'data'
Use require
to Import External Dependencies
To import an external dependency, require
is used. Using the same example above, say, we want to import an external dependency to lib.rb
, we can write:
require 'external_package'