Difference Between Class and Class Instance Variables in Ruby
Nurudeen Ibrahim
Mar 11, 2022
- Difference in Terms of Availability to Class and Instance Methods in Ruby
- Difference in Terms of Inheritance in Ruby
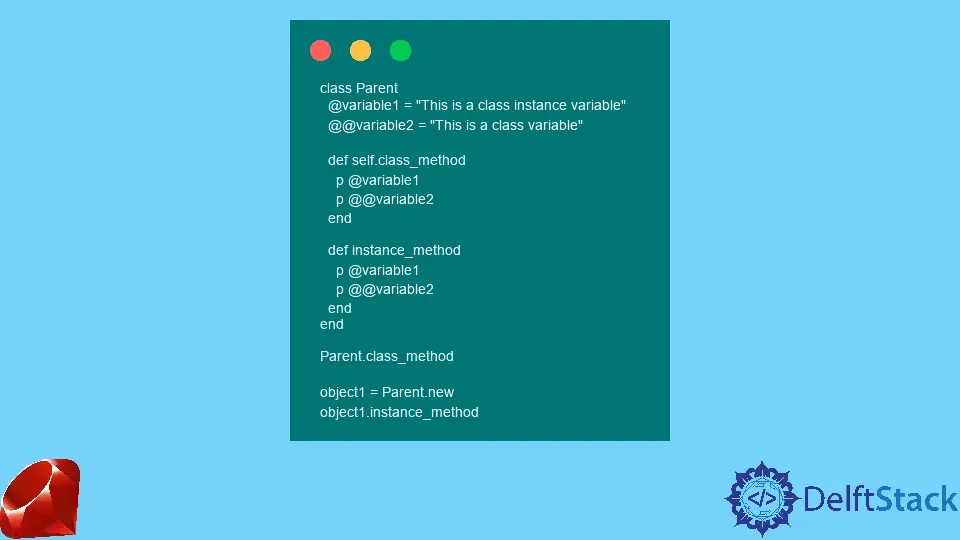
Let’s learn in this tutorial about the difference between the class variable and the class instance variable in Ruby.
Difference in Terms of Availability to Class and Instance Methods in Ruby
Class instance variables are available to only class methods, while class variables are available to both class methods and instance methods.
Example Code:
class Parent
@variable1 = 'This is a class instance variable'
@@variable2 = 'This is a class variable'
def self.class_method
p @variable1
p @@variable2
end
def instance_method
p @variable1
p @@variable2
end
end
Parent.class_method
object1 = Parent.new
object1.instance_method
Output:
"This is a class instance variable"
"This is a class variable"
nil
"This is a class variable"
The first 2-lines of the output above were gotten from Parent.class_method
while the last 2-lines were produced by object1.instance_method
. As we can see, @variable1
was not available in the instance_method
, and as a result, a nil
was produced.
Difference in Terms of Inheritance in Ruby
Class instance variables can not be passed to a child class.
Example Code:
class Parent
@variable1 = 'This is a class instance variable'
@@variable2 = 'This is a class variable'
def self.class_method
p @variable1
p @@variable2
end
def instance_method
p @variable1
p @@variable2
end
end
class Child < Parent
end
Child.class_method
object2 = Child.new
object2.instance_method
Output:
nil
"This is a class variable"
nil
"This is a class variable"