How to Delete File in Ruby
- Understanding File Deletion in Ruby
- Method 1: Using File.delete
- Method 2: Using FileUtils.rm
- Method 3: Handling Exceptions
- Conclusion
- FAQ
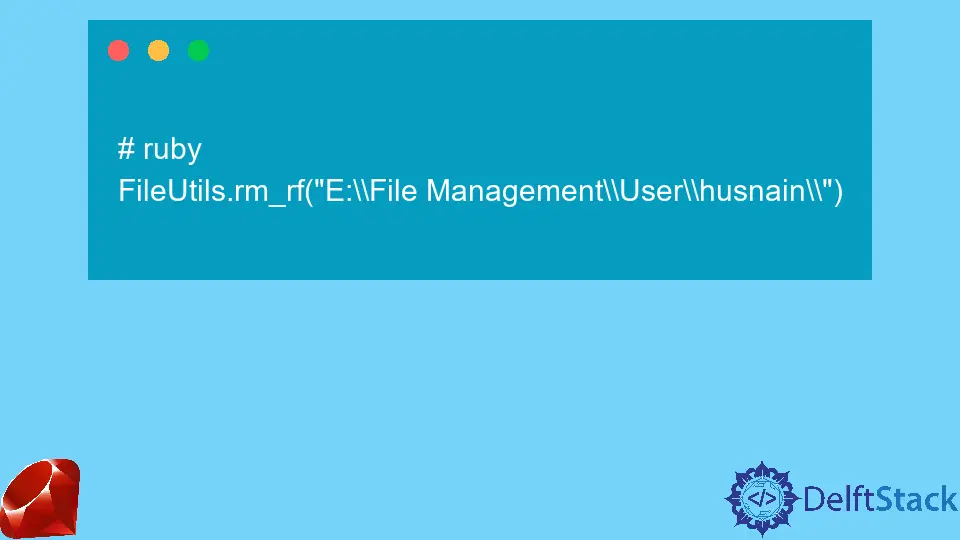
Deleting files in Ruby may seem daunting at first, but with the right approach, it becomes a straightforward task. Whether you’re cleaning up temporary files, managing logs, or simply organizing your project, knowing how to delete files efficiently is crucial.
In this tutorial, we’ll delve into various methods to delete files using Ruby, providing you with clear code examples and detailed explanations. By the end of this guide, you’ll be equipped with the knowledge to handle file deletions in your Ruby applications confidently. Let’s get started!
Understanding File Deletion in Ruby
Before we jump into the code, it’s essential to understand how file deletion works in Ruby. The Ruby programming language provides several built-in methods to manipulate files, including deleting them. The most common method to delete a file is File.delete
, which removes the specified file from the filesystem. This method is straightforward and effective, but there are other approaches you might find useful as well.
Method 1: Using File.delete
The simplest way to delete a file in Ruby is by using the File.delete
method. This method takes the file path as an argument and removes the file from your system. Here’s a basic example to illustrate this:
file_path = "example.txt"
File.delete(file_path)
Output:
0
In this example, we define the variable file_path
with the name of the file we want to delete. The File.delete
method is then called with this variable as an argument. If the file is successfully deleted, it returns the number of files deleted, which in this case is 0
if no error occurs. If the file does not exist, Ruby will raise an exception.
Using File.delete
is a straightforward approach, but you should always ensure that the file exists before attempting to delete it. You can do this using the File.exist?
method to check if the file is present before deletion, which can help avoid unnecessary errors in your application.
file_path = "example.txt"
if File.exist?(file_path)
File.delete(file_path)
else
puts "File not found."
end
Output:
File not found.
This code snippet first checks if the file exists. If it does, it proceeds to delete it; otherwise, it displays a message indicating that the file was not found. This practice enhances the robustness of your code and improves user experience.
Method 2: Using FileUtils.rm
Another effective way to delete files in Ruby is by utilizing the FileUtils
module, specifically the FileUtils.rm
method. This method offers more flexibility and can be particularly useful when dealing with multiple files or directories. Here’s how to use it:
require 'fileutils'
file_path = "example.txt"
FileUtils.rm(file_path)
Output:
0
In this example, we first require the fileutils
library, which provides various methods for file manipulation. The FileUtils.rm
method is then called with the file path, effectively deleting the specified file. Similar to File.delete
, it returns the number of files deleted.
One of the advantages of using FileUtils.rm
is its ability to delete files in bulk. You can pass an array of file paths to delete multiple files at once. Here’s an example:
require 'fileutils'
files_to_delete = ["example1.txt", "example2.txt"]
FileUtils.rm(files_to_delete)
Output:
0
This code snippet demonstrates how to delete multiple files using FileUtils.rm
. It’s a handy feature when you need to clean up several files simultaneously, making your code cleaner and more efficient.
Method 3: Handling Exceptions
When working with file deletion, it’s crucial to handle exceptions gracefully. Ruby provides a way to catch errors using begin-rescue
blocks. Here’s how you can implement this:
file_path = "example.txt"
begin
File.delete(file_path)
puts "File deleted successfully."
rescue Errno::ENOENT
puts "File not found."
rescue StandardError => e
puts "An error occurred: #{e.message}"
end
Output:
File deleted successfully.
In this example, we wrap the File.delete
method in a begin-rescue
block. If the file is deleted successfully, we print a success message. If the file does not exist, the Errno::ENOENT
exception is caught, and we display an appropriate message. The StandardError
rescue clause catches any other exceptions that may occur, ensuring that your program can handle errors without crashing.
This error handling approach is essential for creating robust applications. It allows you to manage unexpected situations gracefully, providing users with clear feedback on what went wrong.
Conclusion
In this tutorial, we explored various methods to delete files in Ruby, including using File.delete
, FileUtils.rm
, and effective error handling techniques. Understanding how to manipulate files is a fundamental skill in Ruby programming, and these methods will help you manage your files efficiently. Whether you’re deleting a single file or multiple files at once, Ruby provides the tools necessary to handle these tasks seamlessly. Armed with this knowledge, you can confidently manage file deletions in your Ruby applications.
FAQ
-
How do I check if a file exists before deleting it?
You can use theFile.exist?
method to check if a file exists before attempting to delete it. -
What happens if I try to delete a file that doesn’t exist?
If you attempt to delete a non-existent file, Ruby will raise anErrno::ENOENT
exception. -
Can I delete multiple files at once in Ruby?
Yes, you can use theFileUtils.rm
method and pass an array of file paths to delete multiple files simultaneously. -
Is there a way to handle errors when deleting files in Ruby?
Yes, you can use abegin-rescue
block to handle exceptions gracefully when deleting files.
- What library do I need to include for using FileUtils?
You need to require thefileutils
library to use theFileUtils
module in Ruby.