How to Convert a String to Lowercase or Uppercase in Ruby
Nurudeen Ibrahim
Feb 02, 2024
Ruby
Ruby String
Ruby Method
-
Convert a String to Uppercase Using the
upcase
Method -
Convert a String to Lowercase Using the
downcase
Method
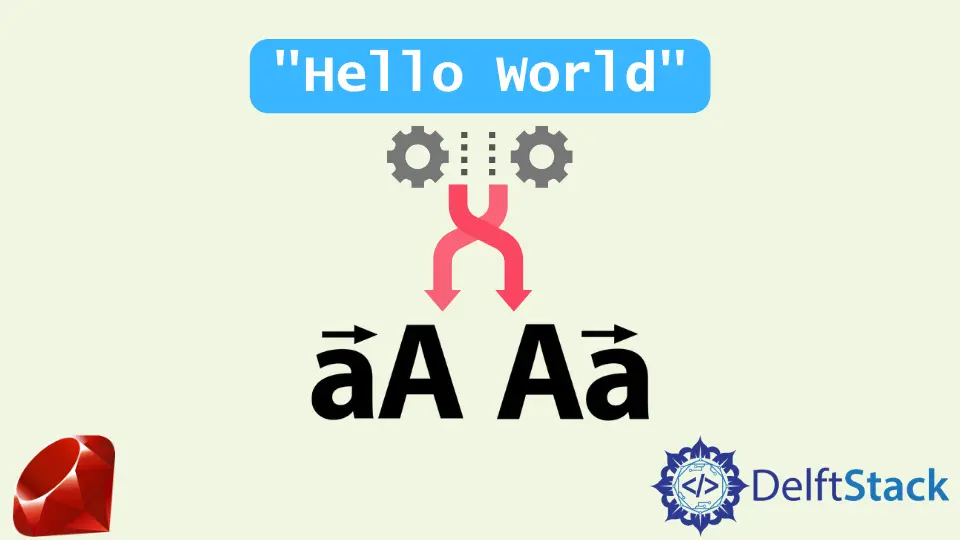
Converting a string from one case to another is a common task in Programming, and Ruby provides two methods that help with that, the upcase
and the downcase
.
Convert a String to Uppercase Using the upcase
Method
str = 'Hello World'
puts str.upcase
Output:
HELLO WORLD
Convert a String to Lowercase Using the downcase
Method
Example Code:
str = 'Hello World'
puts str.downcase
Output:
hello world
It’s worth mentioning that the upcase!
and downcase!
methods work the same way as upcase
and downcase
respectively but instead mutate the original string.
str_1 = 'Hello World'
str_2 = 'Hello World'
puts str_1.upcase!
puts str_1
puts str_2.downcase!
puts str_2
Output:
HELLO WORLD
HELLO WORLD
hello world
hello world
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Related Article - Ruby String
- Literal for Double-Quoted Strings in Ruby
- How to Combine Array to String in Ruby
- How to Get Substring in Ruby
- Difference Between puts and print in Ruby
- How to Compare Strings in Ruby
- How to Capitalize String in Ruby