How to Compare Strings in Ruby
Nurudeen Ibrahim
Feb 02, 2024
- Compare Strings Using the Double Equal Operator in Ruby
-
Compare Strings Using the
eql?
Method in Ruby
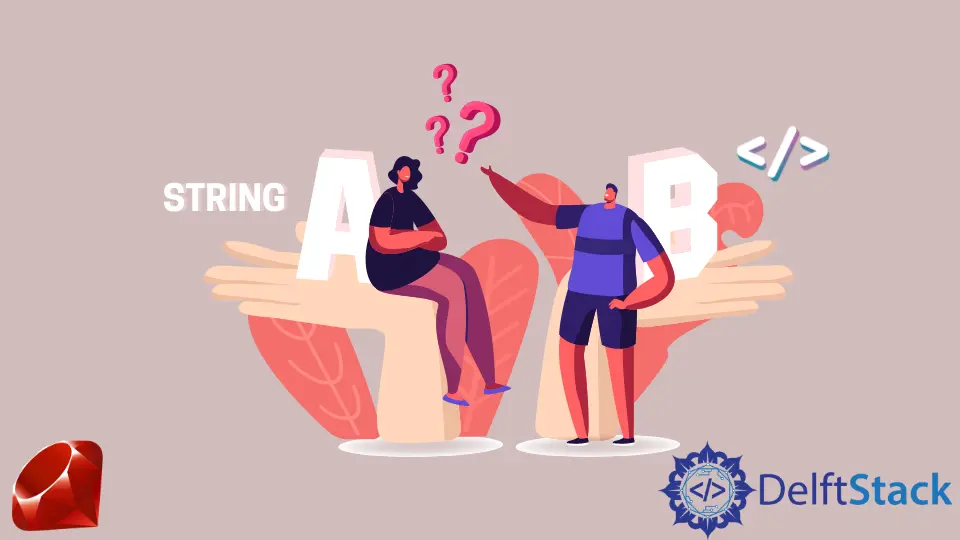
Comparing strings and other objects is a typical operation in Ruby, and below are the two main methods of doing that.
Compare Strings Using the Double Equal Operator in Ruby
The ==
operator is the most common way of comparing objects such as strings in Ruby.
Example code:
str1 = 'John'
str2 = 'John'
str3 = 'Doe'
str4 = 'Dou'
puts str1 == str2
puts str3 == str4
Output:
true
false
Compare Strings Using the eql?
Method in Ruby
Using eql?
is another common method used to compare strings.
Example code:
str1 = 'John'
str2 = 'John'
str3 = 'Doe'
str4 = 'Dou'
puts str1.eql? str2
puts str3.eql? str4
Output:
true
false