call() and send() in Ruby
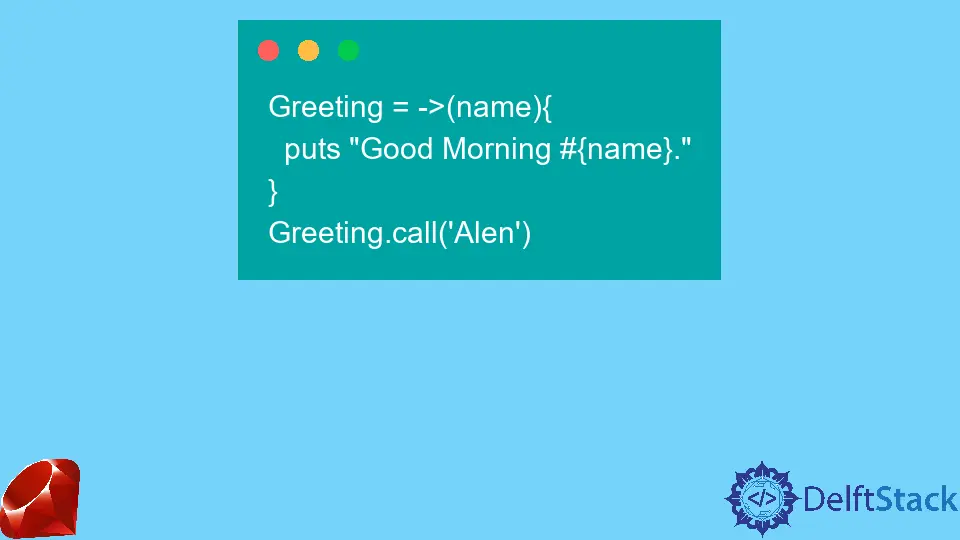
Calling a method or object is a common operation for any object-oriented programming language. Because in object-oriented architecture, the whole system is divided into classes and methods with different properties.
In Ruby, there are multiple ways to call a method or object. You can use either call()
or send()
, but both will provide almost the same result.
In this article, we will discuss the use of call
and send
in Ruby. We also look at an example relevant to the topic to make it easier.
Use call()
in Ruby
In our example below, we will demonstrate how we can use call
. Below shared a simple example that we can take a look at.
Greeting = lambda { |name|
puts "Good Morning #{name}."
}
Greeting.call('Alen')
In the example above, we call through the object Greeting
. After that, it will call its default method.
After running the above example, you will get the below output.
Good Morning Alen.
Use send
in Ruby
In our example below, we will demonstrate how we can use send
. Below shared a simple example that we can take a look at.
def Greeting(name)
puts "Good Morning #{name}."
end
send :Greeting, 'Alen'
In our above example, we first created a method named Greeting()
that takes a string as a parameter.
After running the above example, you will get the below output.
Good Morning Alen.
Both of the methods provide you with the same result. The main difference between call
and send
is that the call
has never been defined on object
, and the send
can be defined for everything.
Please note that all the code this article shares is written in Ruby.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn