How to Use the call() Method in Ruby
- Understanding Procs and Lambdas
-
Using the
call()
Method with Procs -
Using the
call()
Method with Lambdas - Practical Applications of the call() Method
- Conclusion
- FAQ
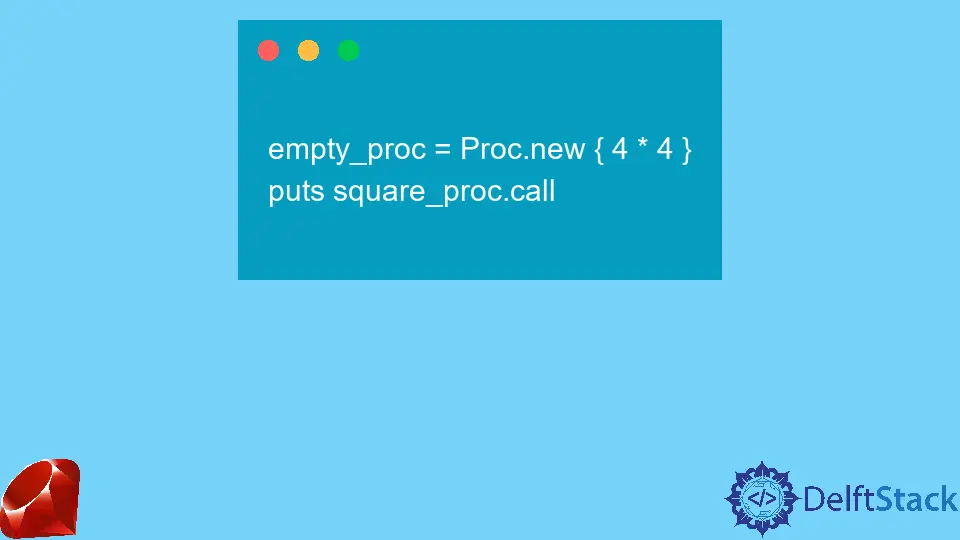
Ruby is an elegant programming language known for its simplicity and productivity. One of the powerful features in Ruby is the call
method, which allows you to invoke a proc or lambda.
This article will guide you through the nuances of using the call
method in Ruby, providing you with practical examples and insights. Whether you’re a beginner or an experienced developer, understanding how to leverage the call
method can enhance your coding skills and improve your Ruby projects. Let’s dive into this essential feature and explore how it can be utilized effectively.
Understanding Procs and Lambdas
Before we delve into the call
method, it’s essential to understand what procs and lambdas are in Ruby. Both are types of closures that encapsulate a block of code, allowing you to pass them around like variables. However, they behave slightly differently.
A proc can take any number of arguments and will not throw an error if you provide fewer arguments than expected. Conversely, a lambda is more strict; it requires the exact number of arguments and will raise an error if the count does not match.
Here’s how you can create a proc and a lambda:
my_proc = Proc.new { |x| x * 2 }
my_lambda = lambda { |x| x * 2 }
Output:
No output
In this example, my_proc
and my_lambda
are both defined to double a given number. Understanding these differences is crucial when using the call
method, as it will influence how you handle arguments.
Using the call()
Method with Procs
The call
method is a straightforward way to execute the code contained within a proc. You can invoke it by calling the proc variable followed by .call
and passing any required arguments.
Here’s a simple example:
result = my_proc.call(5)
puts result
Output:
10
In this snippet, we call my_proc
with an argument of 5
. The proc doubles the number, and the output is 10
. The call
method makes it easy to execute the proc’s code without needing to specify the block syntax. This can be particularly useful in scenarios where you want to encapsulate behavior and execute it dynamically.
Using the call()
Method with Lambdas
Lambdas are invoked in a similar manner to procs, but remember that they enforce strict argument handling. If you pass an incorrect number of arguments, a ArgumentError
will occur. Here’s how you can use the call
method with a lambda:
result_lambda = my_lambda.call(5)
puts result_lambda
Output:
10
In this case, calling my_lambda
with 5
also produces 10
. If you were to call it with no arguments or more than one, you’d encounter an error:
# Uncommenting the line below would raise an error
# my_lambda.call
Output:
ArgumentError: wrong number of arguments (given 0, expected 1)
Using the call
method with lambdas allows you to maintain strict control over the arguments, ensuring that your code behaves predictably.
Practical Applications of the call() Method
The call
method is not just a theoretical concept; it has practical applications in Ruby programming. One common use case is in event handling or callbacks. For example, you might want to execute a block of code when a certain condition is met. Here’s a simple illustration:
def execute_callback(callback)
callback.call("Hello, World!")
end
execute_callback(my_proc)
Output:
Hello, World!
In this example, the execute_callback
method takes a proc as an argument and invokes it using the call
method. This pattern is common in Ruby applications, especially in frameworks like Rails, where callbacks are essential for handling various events.
Conclusion
The call
method in Ruby is a powerful tool for executing procs and lambdas, allowing for flexible and dynamic code execution. By understanding how to use this method effectively, you can enhance your programming skills and create more robust Ruby applications. Whether you’re working with simple calculations or complex event handling, mastering the call
method will undoubtedly benefit your coding journey. So go ahead, experiment with procs and lambdas, and see how the call
method can elevate your Ruby programming experience.
FAQ
-
What is the difference between a proc and a lambda in Ruby?
A proc is more flexible with arguments and does not raise an error if the wrong number is passed, while a lambda enforces strict argument checks. -
Can I use the call() method with regular methods in Ruby?
No, thecall
method is specific to procs and lambdas. For regular methods, you simply invoke them by name. -
What happens if I call a lambda with fewer arguments than required?
You will receive an ArgumentError indicating that the wrong number of arguments was provided. -
Are there any performance differences between procs and lambdas?
Generally, there are negligible performance differences, but lambdas may be slightly faster due to their stricter argument handling. -
How can I pass multiple arguments to a proc using the call() method?
You can simply pass multiple arguments separated by commas, like this:my_proc.call(arg1, arg2)
.