The Binary Left Shift Operator in Ruby
-
the Binary Left Shift Operator (
<<
) in Ruby -
Use the
<<
Operator on an Array in Ruby -
Use the
<<
Operator to Define Class Methods in Ruby -
Use the
<<
Operator inheredoc
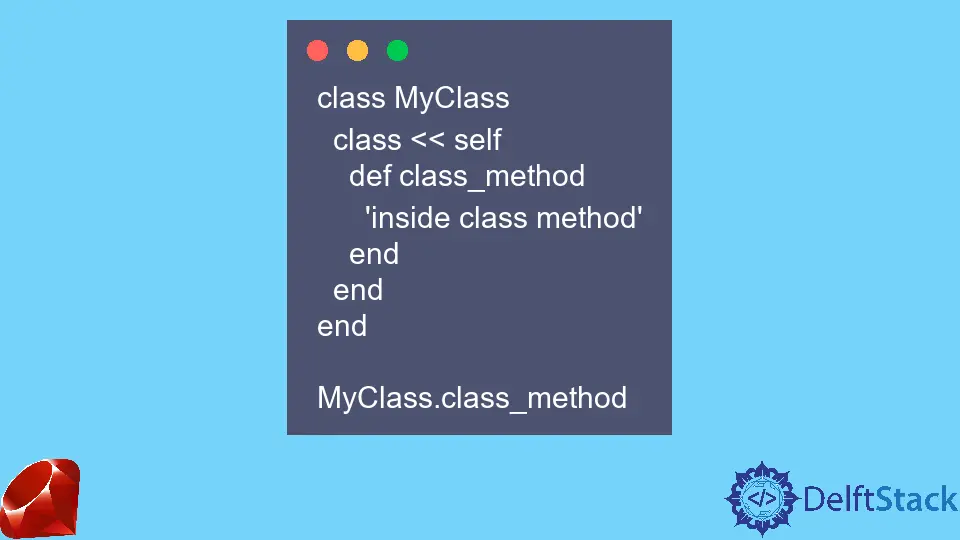
This article will briefly introduce what is <<
in Ruby and where it’s used.
the Binary Left Shift Operator (<<
) in Ruby
The <<
operator is a method of string. It concatenates two strings, and the original string is changed directly.
Code Example:
my_string = 'Hello.'
my_string << ' Nice to meet you!'
puts my_string
Output:
Hello. Nice to meet you!
Use the <<
Operator on an Array in Ruby
Method <<
can be used on an array. It appends an object directly to the end of the original array.
Code Example:
my_array = [1, 2, 3]
my_array << 5
puts my_array
Output:
[1, 2, 3, 5]
This method is similar to the Array#push
method. Note that it can accept any argument, such as another array or hash.
Code Example:
my_array << { a: 1 }
my_array << [6, 7, 8]
puts my_array
Output:
[1, 2, 3, 5, {:a=>1}, [6, 7, 8]]
Use the <<
Operator to Define Class Methods in Ruby
Another popular use of <<
is defining class methods when creating a ruby class.
Code Example:
class MyClass
class << self
def class_method
'inside class method'
end
end
end
MyClass.class_method
Output:
"inside class method"
Use the <<
Operator in heredoc
heredoc
allows us to write a large block of text. The syntax begins with <<
, continues with the document’s name and content, and ends with the document’s name on a separate line.
Example:
<<~MY_DOC
Lorem Ipsum
Donec sollicitudin molestie malesuada.
Proin eget tortor risus. Mauris blandit aliquet elit, eget tincidunt nibh pulvinar a.
MY_DOC
Output:
"Lorem Ipsum\nDonec sollicitudin molestie malesuada.\nProin eget tortor risus. Mauris blandit aliquet elit, eget tincidunt nibh pulvinar a.\n"