How to Use onBlur in JSX and React
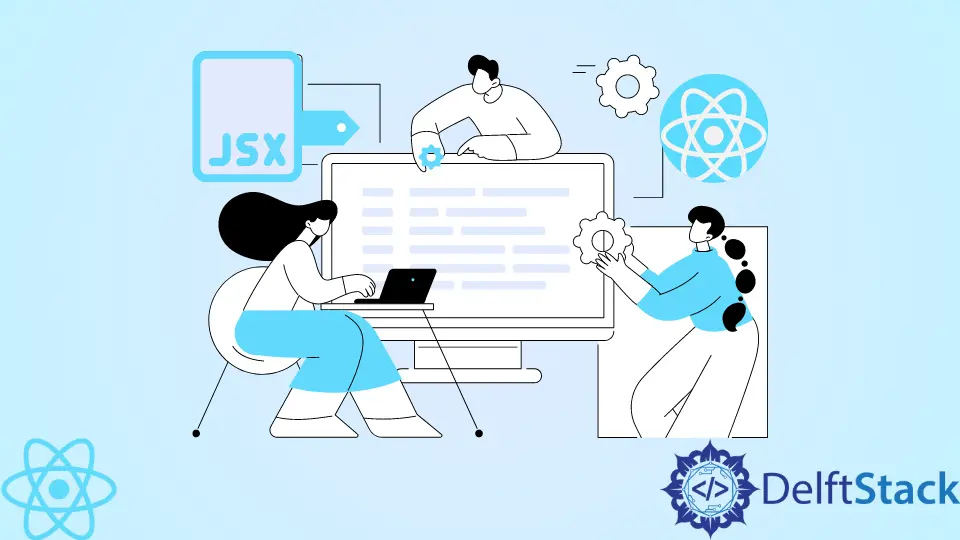
This tutorial will demonstrate implementing the onBlur
function in the React framework.
Use onBlur
in JSX and React
When a form is clicked on to be filled, the onFocus
function becomes active as that part of the framework becomes highlighted. And when the user moves down to the next item on the web page, that form loses focus.
This is when the onBlur
function becomes active. The onBlur
function is one of the best ways to add excellent effects to a web page on the React framework.
In the project below, we will look at the onBlur
function alongside the onFocus
function.
Use onBlur
on a New Project in JSX and React
-
To begin, open the terminal and create a new project by typing,
npx create-react-app new-project
. -
After the new project is created, navigate to the app folder and start a new app using
npm start
. -
Then, open the
App.js
file in the text editor and do some coding.
import React, { useState } from "react";
import "./App.css";
const App: React.FunctionComponent = () => {
const [name, setName] = useState("");
const [isValid, setIsValid] = useState(false);
const [isFocus, setIsFocus] = useState(false);
const [isBlur, setIsBlur] = useState(false);
// Handling input onChange event
const changeHandler = (event: React.ChangeEvent<HTMLInputElement>) => {
setName(event.target.value);
};
// Handling input onFocus event
const focusHandler = (event: React.FocusEvent<HTMLInputElement>) => {
setIsFocus(true);
setIsBlur(false);
// Do something with event
console.log(event);
};
// Handling input onBlur event
const blurHandler = (event: React.FocusEvent<HTMLInputElement>) => {
setIsFocus(false);
setIsBlur(true);
// Validate entered name
if(name.match(/^[a-z][a-z\s]*$/i)){
setIsValid(true);
} else {
setIsValid(false);
}
// Do something with event
console.log(event);
};
return (
<div className="container">
<h3>Please enter your name:</h3>
<input
type="text"
onFocus={focusHandler}
onBlur={blurHandler}
value={name}
onChange={changeHandler}
className="input"
placeholder="Please enter your name"
/>
{isFocus && (
<span className="hint">
Only letters and spaces are acceptable
</span>
)}
{isBlur && !isValid && <p className="error">The name you entered is not valid</p>}
{isBlur && isValid && <p className="success">The name you entered looks good</p>}
</div>
);
};
export default App;
We can add the following code to style and present the web page.
.container {
padding: 50px;
}
.input {
padding: 8px 10px;
width: 300px;
font-size: 18px;
}
.hint {
margin-left: 20px;
}
.error {
color: red;
}
.success {
color: blue;
}
Output:
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn