How to Use Callback With the useState Hooks in React
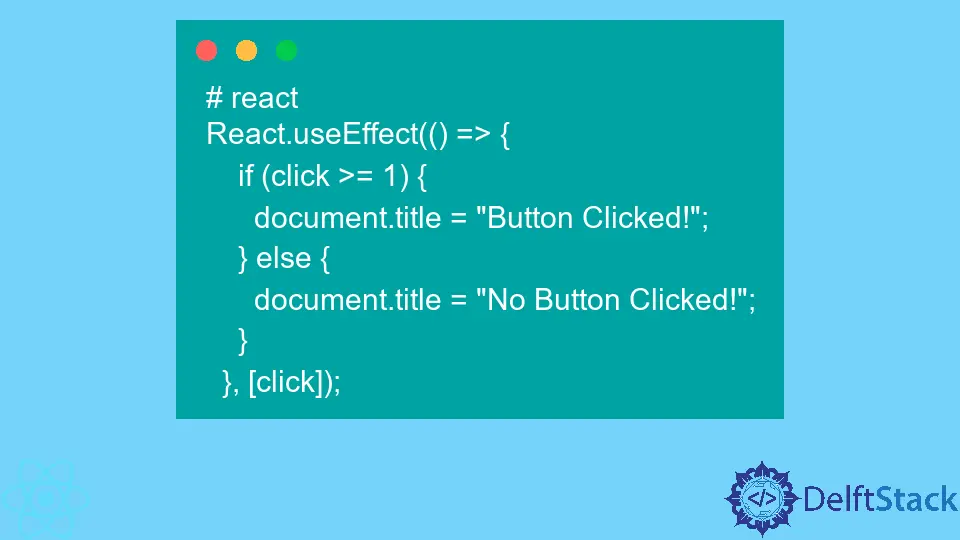
We will introduce useState
and use callback with useState hooks
in React.
useState
in React
useState
function is a built-in hook that can be imported from the React package, which allows us to add state to our functional components.
useState
was introduced from React 16.7
. Using a useState
hook inside a function component allows us to create a piece of state without changing to class components.
To use callback with useState hooks
, we can use useEffect
.
Let’s go through an example. We will create a button; on the button-click page’s title will be changed.
First of all, in app.js
inside the export default function App()
we will declare two constants click
and setClick
using React useState
.
# react
const [click, setClick] = React.useState(0);
Using useEffect
, we will create a function that will check the value of click
. If the click
value is equal to or greater than 1
, it will change document.title
to Button clicked
, and if click
value is smaller than 1
, it will change document.title
to No button clicked
.
# react
React.useEffect(() => {
if (click >= 1) {
document.title = "Button Clicked!";
} else {
document.title = "No Button Clicked!";
}
}, [click]);
Now, we will return the layout.
# react
<div className="App">
<h1>Press button to see changes</h1>
<button type="button" onClick={() => setClick(click + 1)}>
Change Document Title
</button>
</div>
Output:
As seen in the above output, the title of our page is displaying No button clicked. Now let’s check after clicking the button.
Output:
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn