How to Use Hooks in Class Components
- Understanding the Basics of Hooks
- Using State in Class Components
- Managing Side Effects
- Context API in Class Components
- Conclusion
- FAQ
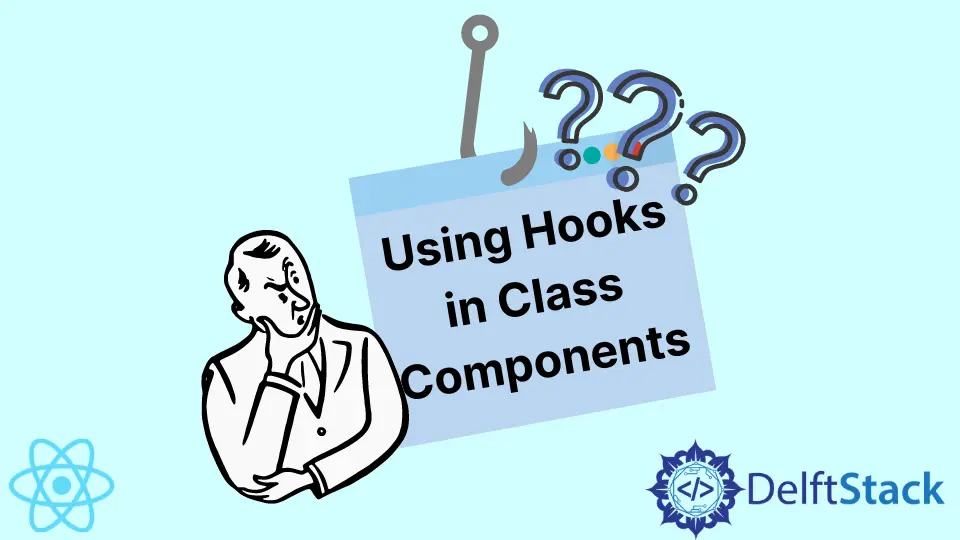
Hooks have revolutionized the way we write React components, primarily enhancing functional components. However, many developers still work with class components and may wonder how to leverage hooks in this context. While hooks are designed for functional components, understanding their principles can help you better structure your class components.
In this article, we will explore strategies for using hooks in class components, making your code cleaner and more efficient. We will also look at how you can integrate hooks to manage state and side effects, which are crucial aspects of any React application. Let’s dive into the world of hooks and class components!
Understanding the Basics of Hooks
Before we delve into practical examples, it’s essential to understand what hooks are. Hooks are functions that let you use state and other React features without writing a class. They enable you to manage component lifecycle events, state, and side effects in a more functional way. The most commonly used hooks include useState, useEffect, and useContext.
While you cannot directly use hooks within class components, you can simulate their behavior. By understanding how hooks operate, you can implement similar functionalities in class components. Let’s explore some methods to achieve this.
Using State in Class Components
One of the primary features of hooks is managing state. In class components, you typically manage state using the this.state
object and this.setState()
. However, you can mimic the behavior of the useState
hook by structuring your state management more effectively.
Here’s how you can manage state in a class component:
import React, { Component } from 'react';
class Counter extends Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
increment = () => {
this.setState(prevState => ({ count: prevState.count + 1 }));
};
render() {
return (
<div>
<h1>Count: {this.state.count}</h1>
<button onClick={this.increment}>Increment</button>
</div>
);
}
}
export default Counter;
Output:
Count: 0
In this example, we create a simple counter component. The state is initialized in the constructor, and we use the increment
method to update the count. This mimics the useState
hook’s behavior by providing a simple way to manage state changes within a class component.
The prevState
parameter in setState
ensures that we are working with the most recent state, which is crucial for accurate state updates, especially in scenarios with multiple updates occurring in quick succession.
Managing Side Effects
Another critical hook is useEffect
, which allows you to perform side effects in functional components. In class components, lifecycle methods serve a similar purpose. You can use componentDidMount
, componentDidUpdate
, and componentWillUnmount
to manage side effects effectively.
Here’s an example of how to handle side effects in a class component:
import React, { Component } from 'react';
class DataFetcher extends Component {
constructor(props) {
super(props);
this.state = { data: null };
}
componentDidMount() {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => this.setState({ data }));
}
render() {
const { data } = this.state;
return (
<div>
{data ? <h1>{data.title}</h1> : <h1>Loading...</h1>}
</div>
);
}
}
export default DataFetcher;
Output:
Loading...
In this example, we fetch data from an API when the component mounts using componentDidMount
. This is analogous to using the useEffect
hook with an empty dependency array, which runs once after the initial render. The state is updated with the fetched data, and the UI reflects this change.
Using lifecycle methods in class components allows you to manage side effects effectively, similar to how you would with hooks in functional components.
Context API in Class Components
The Context API is another powerful feature in React that allows you to share values between components without passing props down manually. The useContext
hook simplifies this process in functional components, but you can achieve the same functionality in class components using Context.Consumer
.
Here’s a quick example of how to use the Context API in a class component:
import React, { Component, createContext } from 'react';
const MyContext = createContext();
class ContextProvider extends Component {
state = { value: 'Hello, World!' };
render() {
return (
<MyContext.Provider value={this.state.value}>
{this.props.children}
</MyContext.Provider>
);
}
}
class ContextConsumer extends Component {
render() {
return (
<MyContext.Consumer>
{value => <h1>{value}</h1>}
</MyContext.Consumer>
);
}
}
export default function App() {
return (
<ContextProvider>
<ContextConsumer />
</ContextProvider>
);
}
Output:
Hello, World!
In this example, we create a context provider that holds a value. The ContextConsumer
class component accesses this value using MyContext.Consumer
. This method allows you to share data across components without prop drilling, similar to how you would use the useContext
hook in a functional component.
Using the Context API in class components can help manage global state effectively, ensuring that your components remain decoupled and maintainable.
Conclusion
While hooks are primarily designed for functional components, understanding their principles can significantly enhance your work with class components. By leveraging state management, side effects, and the Context API, you can create cleaner and more efficient class components. This knowledge not only improves your coding skills but also prepares you for future developments in React as the community continues to embrace hooks. Whether you’re maintaining legacy code or transitioning to functional components, mastering these techniques will make you a more versatile React developer.
FAQ
-
Can I use hooks in class components?
You cannot directly use hooks in class components, but you can achieve similar functionality using lifecycle methods and state management. -
What are some common hooks in React?
Common hooks include useState, useEffect, and useContext, which manage state, side effects, and context, respectively. -
How do I manage state in a class component?
You manage state in a class component using thethis.state
object and thethis.setState()
method. -
What is the purpose of the Context API?
The Context API allows you to share values between components without prop drilling, making it easier to manage global state. -
Are class components still relevant in React?
Yes, class components are still relevant, especially in legacy codebases, but functional components with hooks are becoming the preferred approach for new development.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn