How to Update React Icon Sizes
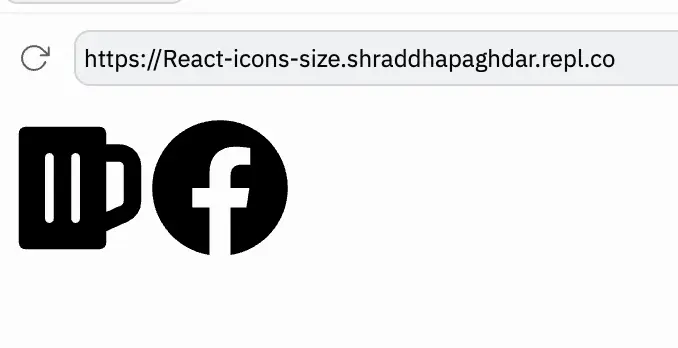
HTML components with icons
are not uncommon when creating complicated apps. The syntax to use icons and set icon sizes is presumably familiar to you if you are familiar with HTML and CSS.
In this article, we’ll learn how to accomplish this in ReactJS by updating icon sizes.
Update React Icon Sizes
Most React developers use JSX
, a unique syntax for creating straightforward component definitions. Since its syntax is similar to HTML, most React engineers favor it.
You’re undoubtedly accustomed to altering icon sizes in HTML by using classes. The size
attribute in JSX
must be set to a number.
An easy definition with the size
attribute would be as follows:
<FaBeer size={24} />
A JavaScript expression that yields an integer can be used to set the size
attribute to that value. It would help if you enclosed the expressions in curly brackets to guarantee they are correctly recognized as JavaScript code.
Let’s look at an illustration below:
import {FaBeer} from 'react-icons/fa';
const sizeValue = 14 * 5;
<FaBeer size={sizeValue} />
<FaFacebook size={sizeValue}></FaFacebook>
In this illustration, we combine a standard icon size
with an integer value that may be dynamically stored in a variable. Our size
attribute will equal the integer 70
after evaluating the equation.
Now, run the above line of code in Replit, which is compatible with React.js. It will display the following outcome. You can also find its demo here.
Output:
You can find more information about react-icons here.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn