The className Attribute in JSX
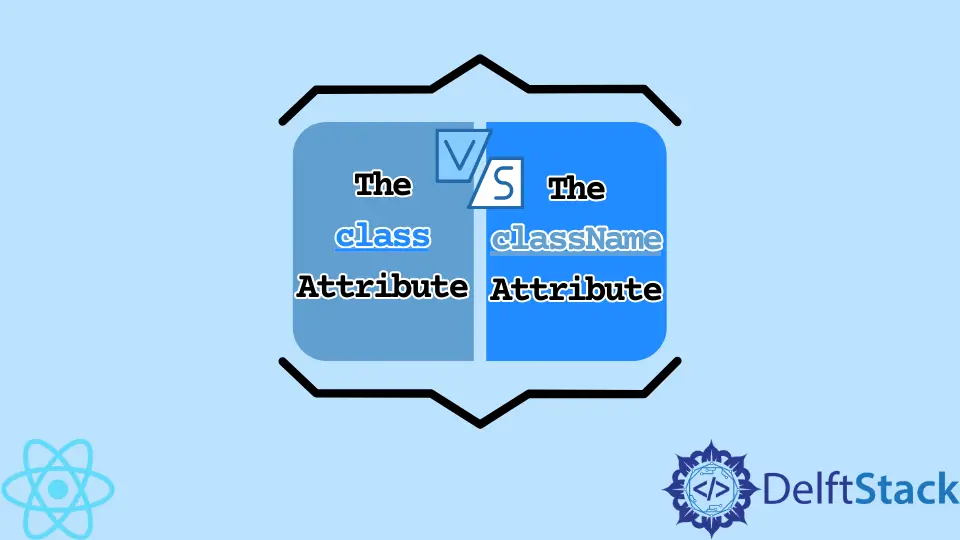
This article will analyze the changes that happen when using the class
and the className
attribute and what differentiates them.
the class
Attribute vs. The className
Attribute in JSX
When working on HTML, we usually use the class
attribute to target an element for styling with CSS.
For example, we create a div
like below:
<div class="body">
<p>
Click me!
</p>
</div>
We are able to target the body element in CSS which is:
.body {
position: absolute;
}
But when it comes to React, the class
attribute performs a different function of creating objects as it is a keyword in JavaScript.
Even though we can use a class
and className
attributes in React, this will mostly lead to contradictions and bugs when browsers, especially older browsers, are reading these codes.
So the best turnaround to this is using the className
attribute instead of class
in React. And the amazing thing is after the codes have been compiled and opened in browsers when inspected, we don’t see className
; instead, we see class
.
And just like in HTML, the className
will be targeted for styling in the CSS like this:
<div className='box'>
<p>
Click me!
</p>
</div>
.box {
background-color: blue;
}
So to avoid complications and correct better codes, it is ideal to use the className
attribute for elements in the React framework.
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn