How to Display SVG Files in React
-
Use the
src
Attribute to Includesvg
in React -
Include Locally Hosted
svg
File With React increate-react-app
2.0
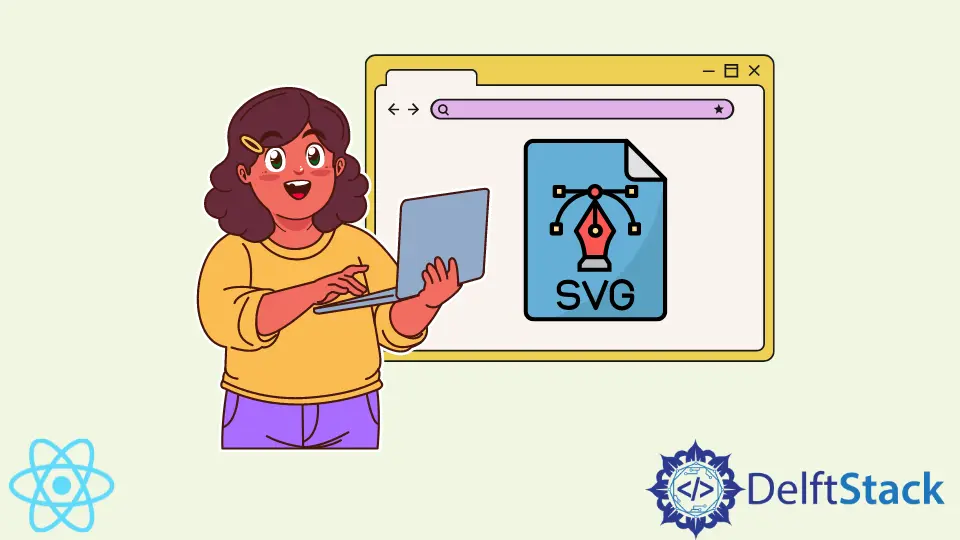
React is often hailed as one of the most popular JavaScript frameworks, but in fact, it’s just a library for building user interfaces. Like any other web app, React apps that feature images, videos, and other visual content are more engaging than others.
These days, more and more developers are using .svg
icons to display important visuals like logos.
Use the src
Attribute to Include svg
in React
There are multiple ways to feature .svg
files in a React application. This article discusses two ways to include .svg
visuals in your React app.
One way to feature visual files with an svg
file extension is to use the src
attribute of the <img>
element. Let’s look at a sample that features a .svg
file.
export default function App() {
return (
<div className="App">
<img
src={"https://svgshare.com/i/e77.svg"}
style={{ width: 50, height: 50 }}
></img>
</div>
);
}
As you can see, the source file is in svg
format, but if you look on CodeSandbox, this code works and indeed displays the visual as it should.
However, displaying these files as <img>
elements means that they will be treated as normal images and lose unique features of svg
files.
As a result, featuring an svg
image this way might remove some of its unique properties. It’s better to host them locally.
Include Locally Hosted svg
File With React in create-react-app
2.0
The updated version of the create-react-app
package includes the necessary loaders and allows you to import locally stored .svg
assets and use them without sacrificing any of their properties.
Below is an example of using the import
statement to feature .svg
files.
import { ReactComponent as LogoSvg } from './assets/Logo.svg';
We specify the relative path to the svg
file and import ReactComponent
named export, although we use the as
statement to reference it as LogoSvg
in our application.
It would be best if you kept in mind that the ReactComponent
name is not optional, and it’s necessary to import svg
files in create-react-app
.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn