How to Show and Hide Element in React
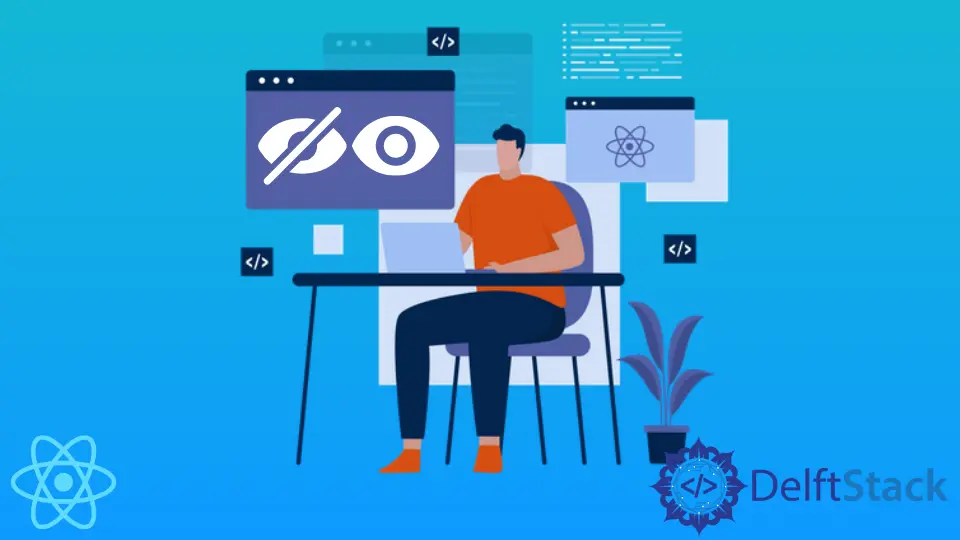
useState
and useEffect
hooks are part of React 16.8+, which provides functional components the same as class-based components. We will introduce these functions in this article.
When developing a commercial application, we need to hide some data based on user roles or conditions. We will give an example of showing and hiding data based on conditions.
Use useState
and useEffect
to Hide Message in React
First, we will define constants showMessage
and setShowMessage
, and set its useState
to true. Using useEffect
, we will change the state to false after 10 seconds.
# react
import React, { useState, useEffect } from "react";
export default function App() {
const [showMessage, setShowMessage] = useState(true);
useEffect(() => {
setTimeout(function () {
setShowMessage(false);
}, 10000);
}, []);
return (
<div className="App">
{showMessage && <h1>This text will show!</h1>}
</div>
);
}
Output:
As you can see, the text is displayed for only 10 seconds and disappears after.
If we want to hide the element whenever a button is clicked, we will create a button that will change the state of the message that will hide it and show it on button clicks.
# react
<button onClick={() => setShowMessage(!showMessage)}>Show Text</button>
Let’s check how it works.
Output:
In this way, we can easily hide and display elements or components in react using useEffect
and useState
.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn