Client-Side vs. Server-Side Rendering in React
- Client-Side Rendering in React
- Server-Side Rendering in React
- Client-Side vs. Server-Side Rendering in React
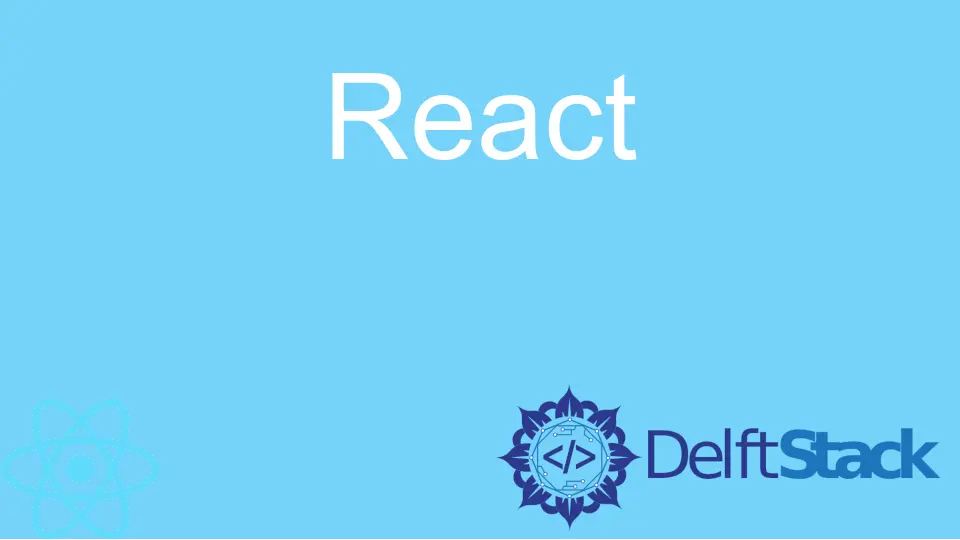
Traditionally, web pages used to be rendered on the server, but with the emergence of JavaScript-based frameworks like React, client-side rendering has gained momentum. These days, web applications can be rendered on the server, in the browser (client-side), or use some combination of the two.
Client-Side Rendering in React
React framework is primarily used to build applications rendered on the client-side. Most of the tutorials you see online describe the process of building single-page applications.
Tutorials in React docs follow this pattern, and so does create-react-app
, a simple package that allows you to start building React applications quickly.
React applications rendered on the client-side are decoupled from the server. The server only renders initial HTML, which is essentially an empty page.
Then, React renders interactive elements on the client-side to fill the page with content, visuals, and everything else that makes up the page. For example, Netflix uses React heavily to render interactive elements on the client-side.
Server-Side Rendering in React
You can use React to render the whole page (including texts, visuals, and contents) on the server. When the user visits a website, the server will load the entire HTML page populated with all of its content.
This approach allows web crawlers, such as Google bots, to index the page with all of its content.
However, if the page is completely rendered on the server-side, it can not contain interactivity features. For example, if the Facebook homepage were only rendered on the server, users would not be able to see new notifications without refreshing the page.
Client-Side vs. Server-Side Rendering in React
Most websites like Facebook and Netflix use a combination of both to get the best of both worlds. For instance, if you want your website to be indexed by Google and earn search engine traffic, you should render all of your text content on the server-side.
Client-side applications may look good to the visitors, but bots only see an empty HTML page.
When using the combination of CSR (client-side rendering) and SSR (server-side rendering), the server renders an HTML file with the minimum necessary content. Once the scripts are loaded, you can use the client-side rendering approach to display interactive features.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn