How to Scroll to Top in React
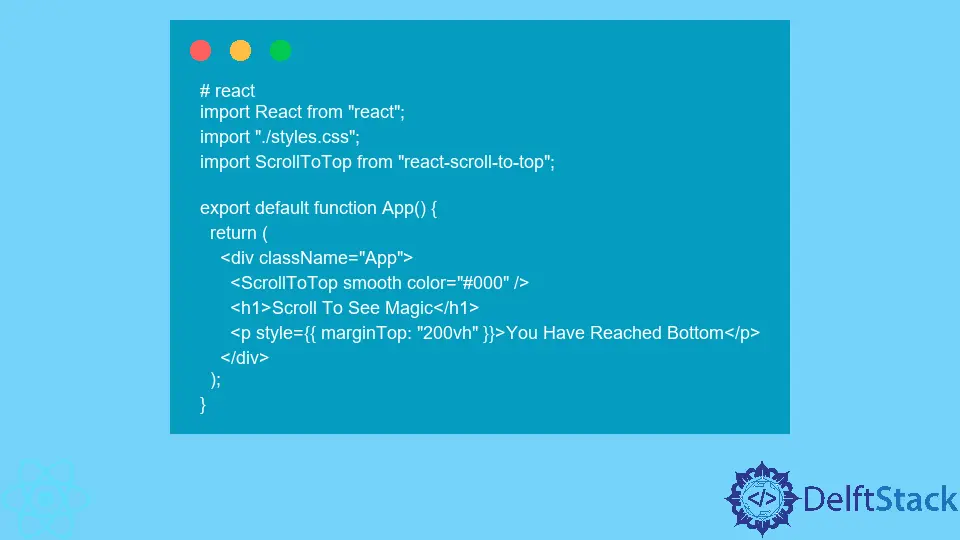
Creating a seamless user experience is crucial in web development, and one common feature that enhances usability is the “scroll to top” button. This feature allows users to quickly return to the top of the page without having to scroll manually, which is especially useful on long pages.
In this tutorial, we will explore how to implement a scroll-to-top button in a React application. We’ll cover different methods, including using hooks and class components, so you can choose the best approach for your project. Whether you’re a beginner or an experienced developer, this guide will provide you with the insights you need to effectively implement this feature.
Method 1: Using React Hooks
React Hooks are a powerful feature that allows you to use state and other React features without writing a class. One of the simplest ways to create a scroll-to-top button is by using the useEffect
and useState
hooks. Here’s how you can do it:
import React, { useEffect, useState } from 'react';
const ScrollToTopButton = () => {
const [isVisible, setIsVisible] = useState(false);
const handleScroll = () => {
if (window.scrollY > 300) {
setIsVisible(true);
} else {
setIsVisible(false);
}
};
const scrollToTop = () => {
window.scrollTo({
top: 0,
behavior: 'smooth'
});
};
useEffect(() => {
window.addEventListener('scroll', handleScroll);
return () => {
window.removeEventListener('scroll', handleScroll);
};
}, []);
return (
<button onClick={scrollToTop} style={{ display: isVisible ? 'block' : 'none' }}>
Scroll to Top
</button>
);
};
export default ScrollToTopButton;
Output:
A button that appears after scrolling down 300 pixels and smoothly scrolls to the top when clicked.
In this code snippet, we start by importing the necessary React hooks. The useState
hook is used to manage the visibility of the button based on the scroll position. The handleScroll
function checks the vertical scroll position (window.scrollY
). If it’s greater than 300 pixels, the button becomes visible. The scrollToTop
function uses window.scrollTo
with behavior: 'smooth'
to create a smooth scrolling effect when the button is clicked.
The useEffect
hook is employed to add an event listener for the scroll event and to clean it up when the component unmounts. This ensures that we do not have memory leaks in our application.
Method 2: Using Class Components
If you prefer using class components instead of hooks, you can achieve the same functionality with a slightly different approach. Here’s how to implement a scroll-to-top button in a class component:
import React, { Component } from 'react';
class ScrollToTopButton extends Component {
constructor(props) {
super(props);
this.state = {
isVisible: false
};
this.handleScroll = this.handleScroll.bind(this);
this.scrollToTop = this.scrollToTop.bind(this);
}
componentDidMount() {
window.addEventListener('scroll', this.handleScroll);
}
componentWillUnmount() {
window.removeEventListener('scroll', this.handleScroll);
}
handleScroll() {
if (window.scrollY > 300) {
this.setState({ isVisible: true });
} else {
this.setState({ isVisible: false });
}
}
scrollToTop() {
window.scrollTo({
top: 0,
behavior: 'smooth'
});
}
render() {
return (
<button onClick={this.scrollToTop} style={{ display: this.state.isVisible ? 'block' : 'none' }}>
Scroll to Top
</button>
);
}
}
export default ScrollToTopButton;
Output:
A button that appears after scrolling down 300 pixels and smoothly scrolls to the top when clicked.
In this class component, we set up the same functionality as in the hooks example. The constructor initializes the state and binds the methods. The componentDidMount
lifecycle method is used to add the scroll event listener, while componentWillUnmount
removes it to prevent memory leaks. The handleScroll
method updates the visibility state based on the scroll position, and the scrollToTop
method smoothly scrolls the page to the top when the button is clicked.
Conclusion
Implementing a scroll-to-top button in React is a straightforward process that significantly enhances user experience. Whether you prefer using functional components with hooks or class components, both methods are effective and easy to implement. By following the examples provided in this tutorial, you can create a smooth and responsive scroll-to-top feature that will make navigating your application more user-friendly. So, go ahead and try it out in your next React project!
FAQ
-
How do I customize the scroll-to-top button?
You can customize the button’s appearance by applying CSS styles or using a library like styled-components. -
Can I change the scroll threshold from 300 pixels?
Yes, you can easily adjust the value in thehandleScroll
function to any pixel value that suits your needs. -
Is it possible to make the button fade in and out?
Absolutely! You can use CSS transitions to create a fade effect when the button appears or disappears. -
Does the scroll-to-top button work on mobile devices?
Yes, the button works on mobile devices as long as the browser supports thescrollTo
method. -
Can I add an icon to the scroll-to-top button?
Yes, you can include an icon by using an icon library like Font Awesome or by adding an image tag inside the button.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn